Java Loop
Loop execute block of code repeatedly till the condition is true. Java provides three kind of loop statement:
- While Loop
- Do While Loop
- For Loop
While Loop:
While loop repeats till the given Boolean condition is true.
As shown in the below diagram, the statement will run till the condition check is true.

Syntax:
while (condition true) {
// block of code
}
Example:
Here the while loop will run till the condition is true for x which is less than 3.
public class WhileLoop {
static int x = 1;
public static void main(String[] args) {
while(x < 3) {
System.out.println("x = "+x);
x++;
}
}
}OUTPUT:
x = 1
x = 2
Do While Loop:
Do While loop is like while loop with one minor difference. Do while loop always runs once before checking the condition.

Syntax:
do {
// Block of code
} while(condition);
Example:
As mentioned earlier, the do statement or block of code will always execute once before checking the condition is true or false.
static int x = 5;
public static void main(String[] args) {
do {
System.out.println("x = "+x);
x++;
} while(x < 3);
}OUTPUT:
x = 5
For Loop:
For loop is quite different from while and do while loop. In single line of statement, we define the initialization, condition (true or false), increment/decrement.

Syntax:
For (initialization; condition; increment/decrement) {
// Block of code
}
Example:
public static void main(String[] args) {
for(int i = 0 ; i < 2 ; i++) {
System.out.println("i = " + i);
}
}OUTPUT:
i = 0
i = 1
Initialization: The int i = 0 is the condition for loop initialization.
Condition: This will check if the condition is true to run block or code inside for loop. The false condition will terminate the loop.
Increment/decrement: This will update the variable for next iteration.
Enhanced for loop:
It provides a simple way to iterate through the loop. This provides a unique way to iterate over array and list of objects. While iteration through array or list, code will not allow us to update the value. The array or list value can be access sequentially after running loop without knowing the index.
Syntax:
For(datatype a : array /object_list) {
// Block of code
}
Example:
public static void main(String[] args) {
String array[] = {"one", "two", "three"};
for(String str : array) {
System.out.println(str);
}
}OUTPUT:
one
two
three
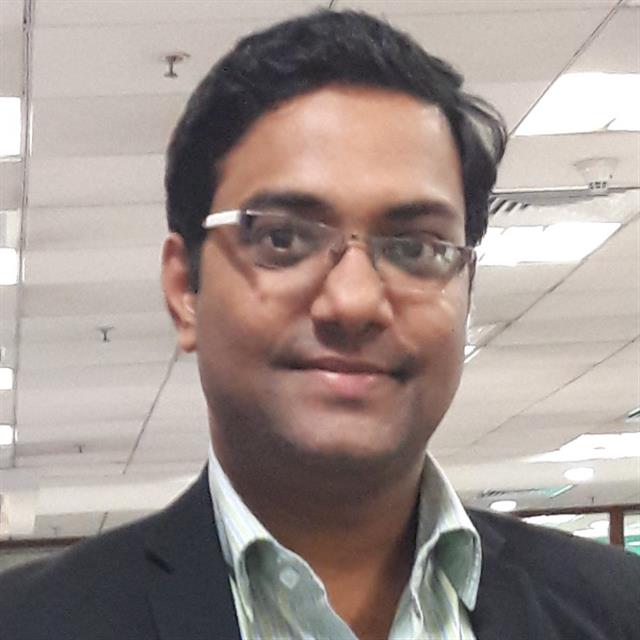
Imran Khan, Adobe Community Advisor, certified AEM developer and Java Geek, is an experienced AEM developer with over 12 years of expertise in designing and implementing robust web applications. He leverages Adobe Experience Manager, Analytics, and Target to create dynamic digital experiences. Imran possesses extensive expertise in J2EE, Sightly, Struts 2.0, Spring, Hibernate, JPA, React, HTML, jQuery, and JavaScript.