Inheritance and Diamond Problem
To understand the diamond problem, we first need to understand inheritance.
Inheritance is a process to acquire or inherit one class (base or parent) of attributes or behavior in to another class (child or derived).
Inheritance is all about making a relation in between parent and child class.
Types of Inheritance:
- Single Inheritance
- Multiple Inheritance
- Hybrid Inheritance
- Hierarchical Inheritance
- Hybrid Inheritance
Child class is the one which inherits the parent class to access its data variable and members from parent class.
Let’s discuss about various types of inheritance supported by Python:
Single Inheritance
As mentioned in below diagram, class B is subclass of parent class A.
class B inheriting everything from class A.

Syntax:
Below is the syntax for single inheritance:
# class A is a parent class with printName() method
class A:
#Bock of code
# class B is inheriting class A and all its data variables and
Class B(A):
pass
Example:
# class A is a parent class with printName() method
class A:
def __init__(self, name):
self.name = name
def printName(self):
print(self.name);# class B is inheriting class A and all its data variables and functions.
class B(A):
pass
obj= B("test");# class B object is accessing Class A method using inheritance.
obj.printName();
OUTPUT:
test
we have written pass keyword to avoid empty class error for class B. As empty class and function is not allowed in Python.
Multiple Inheritance
Multiple class inheritance is all about a single class inheriting multiple classes.
In below diagram, class A is inheriting both class B and class C at the same time showing multiple inheritance.

Syntax:
# class A is a parent class with printName() method
class B:
#Bock of code# class B is inheriting class A and all its data variables and
Class C:
#Bock of code# class C is inheriting class B and all its data variables and
Class A(B, C):
#Bock of code
Example:
In the below example, class A in inheriting both class B and class C.
class A has access to all the data members and functions of class B and class C.
In below multilevel inheritance there is no relation in between class B and class C.
# class B is a parent class with printName() method
class B:
def printB(self):
print("Inside B");
# class Cis inheriting class A and all its data variables and
class C:
def printC(self):
print("Inside C");
# class A is inheriting class B and class C at the same time.
class A(C,B):
def printA(self):
print("Inside A");
obj = A();
obj.printA();
obj.printB();
obj.printC();OUTOUT:
Inside A
Inside B
Inside c
Multilevel Inheritance
As mentioned in below diagram, class B is subclass of super class A.
Class B is inheriting everything from Class A.
Below is the chain of inheritance which is called as multilevel inheritance where class c inherits class B and class B inherits class A. In this case indirectly class C is inheriting class A.

Syntax:
# class A is a parent class with printName() method
class A:
#Bock of code# class B is inheriting class A and all its data variables and
Class B(A):
#Bock of code# class C is inheriting class B and all its data variables and
Class C(B):
#Bock of code
Example:
In the below example, class C in inheriting class B and class Bis inheriting extends Class A.
class B has access to all the data members and methods of class A.
Similarly, class C also has access to all the data members and methods of class B.
This means, class C has access to all data members and methods of class A and class B as show in below example.
# class A is a parent class with printName() method
class A:
def printA(self):
print("Inside A");
# class B is inheriting class A and all its data variables and
class B(A):
def printB(self):
print("Inside B");
# class C is inheriting class B and all its data variables and
class C(B):
def printC(self):
print("Inside c");
obj = C();
obj.printA();
obj.printB();
obj.printC();OUTOUT:
Inside A
Inside B
Inside c
Hierarchical inheritance:
When two or more class inherits the same class called as Hierarchical inheritance.
Note: There is not relation in between class B and class C. Accessing of data members and functions will give compile time error.

Syntax:
# class A is a parent class with printName() method
class A:
#Bock of code# class B is inheriting class A and all its data variables and functions
Class B(A):
#Bock of code# class C is inheriting class A and all its data variables and
Class C(A):
#Bock of code
Example:
# class A is a parent class with printName() method
class A:
def printA(self):
print("Inside A");
# class B is inheriting class A and all its data variables and functions.
class B(A):
def printB(self):
print("Inside B");
# class C is inheriting class B and all its data variables and functions.
class C(A):
def printC(self):
print("Inside C");
obj = C();
obj.printA();
obj.printC();# Below method call will give error as there is no relation in between class B and C.
#obj.printB();OUTOUT:
Inside A
Inside C
Hybrid Inheritance
Hybrid Inheritance is all about combination of different inheritance (e.g. multiple and hierarchical) is called as Hybrid.
In below diagram, class B is inheriting class A, class C is inheriting class A, class D is inheriting class B and class D is inheriting class C. This means class D internally or virtually inheriting class A.
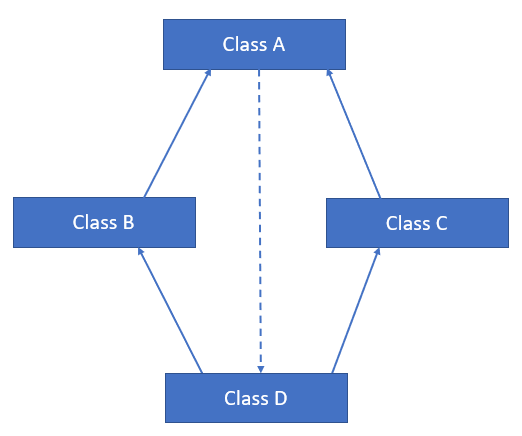
Example:
# class A is a parent class with printA() method
class A:
def printA(self):
print("Inside A");
# class B is inheriting class A
class B(A):
def printB(self):
print("Inside B");
# class C is inheriting class A
class C(A):
def printC(self):
print("Inside C");# class B is inheriting class C and class C
class D(B,C):
def printD(self):
print("Inside D");
obj = D();# class D is internally inheriting class A
obj.printA();obj.printB();
obj.printC();
obj.printD();OUTPUT:
Inside A
Inside B
Inside C
Inside D
Why doesn’t Python have a diamond problem?
The Diamond problem doesn’t exist in Python because it gives preference to the class that gets inherited first. In the following example, “class D(C,B)” denotes that class D inherited class C first, followed by class B. Here, class C functions will take preference over class B.
Example:
# class A is a parent class with printA() method
class A:
def printName(self):
print("Inside A");
# class B is inheriting class A
class B(A):
def printName(self):
print("Inside B");
# class C is inheriting class A
class C(A):
def printName(self):
print("Inside C");
# class C will take priority over class B
class D(C,B):
pass
obj = D();
# while inheriting classes whichever inherited first will take precedence.
obj.printName();OUTPUT:
Inside C
__init()__ and super
Add __init__() method and super keyword in child class:
Please read comments while reading the code below. It will help to understand better.
# class A is a parent class with printName() method
class A:
def __init__(self, name):
self.name = name
def printName(self):
print(self.name);
# class B is inheriting class A
class B(A):
# declaring __init__() method inside class B will stop calling class A __init__() method.
def __init__(self, name):
# use super() function to call parenet class __init_() method.
super().__init__(name)
obj= B("test");
# class B object is accessing Class A method using inheritance.
obj.printName();
OUTPUT:
test
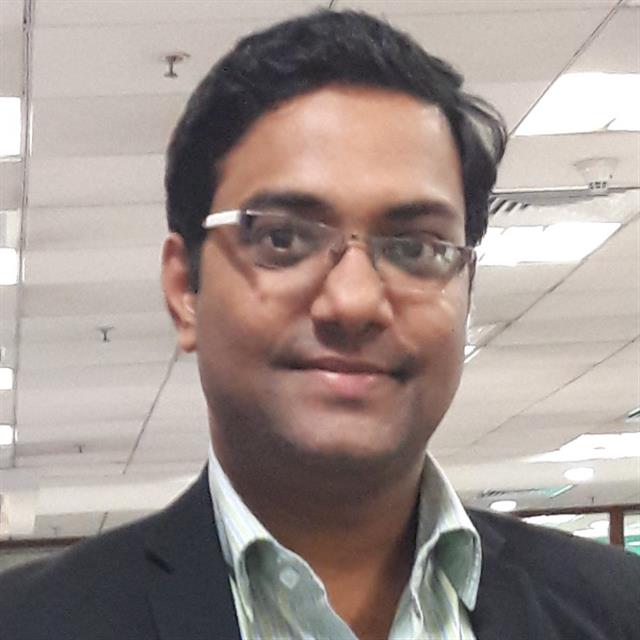
Imran Khan, Adobe Community Advisor, AEM certified developer and Java Geek, is an experienced AEM developer with over 11 years of expertise in designing and implementing robust web applications. He leverages Adobe Experience Manager, Analytics, and Target to create dynamic digital experiences. Imran possesses extensive expertise in J2EE, Sightly, Struts 2.0, Spring, Hibernate, JPA, React, HTML, jQuery, and JavaScript.