Class
Classes and objects are basic building block of Object Oriented programming language. Class is a kind of block that contains various data types and methods. Class is just a blueprint from which objects are created.
Class contains multiple things:
1. variables
2. methods
3. Constructor
Class Creation
To create a class we use class “keyword”
Syntax:
class <class_name>:
# block of code or statements
Example:
Lets try to understand the below example where we have created a class named as Language and a variable named as color with the value red. Create an object as we created obj in below example to access the variable color defined inside the Language class. We can access a color variable with a class object as obj.color
class Language:
color = "red";obj = Language();
print(obj.color);
OUTPUT:
red
__init__() Function
It’s important to understand the role of the __init__() function as it will get called whenever a class intializes. This method comes into the picture to initialise variables or perform some action as soon as the class object initialize.
self represents to the current object of the class and it will always be there as part of function parameter.
Example:
class Language:
def __init__(self, name):
self.name = name;obj = Language("test");
print(obj.name);
OUTPUT:
test
Class Functions
A function is a block of code that performs some action which executes only on calling.
The function declares a comma-separated variable within parentheses called as “parameters.”
Example:
class Language:
def __init__(self, name):
self.name = name;def printName(self):
print(self.name);
obj = Language("test");
obj.printName();
OUTPUT:
test
Delete an object or Property:
The object or property can be deleted using del keyword.
# Delete propert from object.
del obj.name;
#Delete object.
del obj;
pass keyword:
A pass keyword will allow a loop to run an empty statement and pass through. This pass is used to ensure that a block of code is not left empty. This helps us to add a block of code in the future for one of the empty conditions. Leaving a block of code empty under one of the conditions may give a compilation or code quality error by one of the code quality tools.
We can have class with not content and pass helps us to create an empty class. putting pass in an empty class helps us to avoid getting an error on run time.
languages = ["HTML", "CSS", "Java", "Javascript"];for language in languages:
if language == "Java":
pass; # do nothing, just pass through when language is Java.
else:
print(language)
OUTPUT:
HTML
CSS
Javascript
Without pass keyword:
declaring an empty class is giving an error.
class a:OUTPUT:
File “<string>”, line 1
class a:
^
SyntaxError: unexpected EOF while parsing
With pass keyword:
Declaring an empty class with pass keyword is not giving an error as it is not completely empty and having a pass keyword which does nothing.
class a:
passOUTPUT:
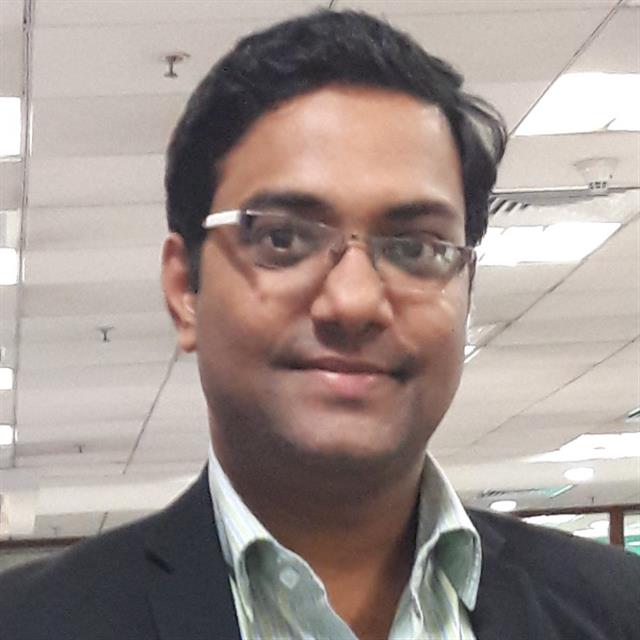
Imran Khan, Adobe Community Advisor, AEM certified developer and Java Geek, is an experienced AEM developer with over 12 years of expertise in designing and implementing robust web applications. He leverages Adobe Experience Manager, Analytics, and Target to create dynamic digital experiences. Imran possesses extensive expertise in J2EE, Sightly, Struts 2.0, Spring, Hibernate, JPA, React, HTML, jQuery, and JavaScript.