Data Types
This will provide a complete knowledge in detail about data and its type with example.
To understand variable, let’s first discuss about data type.
Variables are used to store value.
name = “John”
num = 2
As mentioned above, name and num we are using to store John and 2 as a value. Here, name and num are variables. John and 2 are the values. We can give any customized user-defined name to a variable and assign any type of value as a number, character, word, decimal, etc.
In Python, there is no concept to declare data type while assigning value. Python will decide the variable data type or type of the data as soon as we assign the value to a variable.
name = “John”
num = 2
In above example, name is type of str and num is type of int.
Data Types
There are different type of Python data types:
- Numeric -> int, float, complex
- String -> str
- Sequence -> list, tuple
- set
- map -> dictionary
type(object)
This will return and help us to know the type of data.
Example
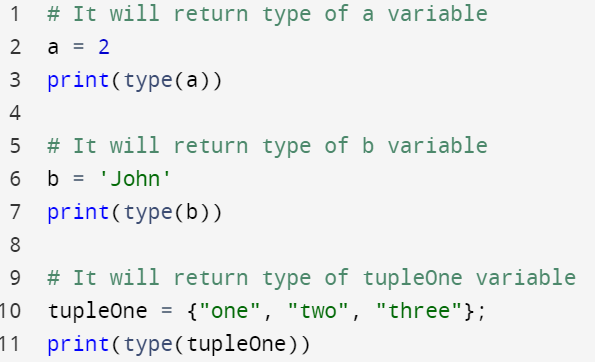
OUTPUT:

Numeric
Numeric data type majorly get divided into int, float and complex.
int: This used to store any length integer or numeric value.
float: This used to store floating precision number up to 15 decimal places.

OUTPUT:

String (str)
str is a data type used to store String or sequence of characters.

OUTPUT

Sequence Variable
Sequence variable is used to store data with multiple values.
- List → languages = [“CSS”, “HTML”, “Java”]
List is declare by using square bracket, it is ordered, allow duplicate values, changeable(allow update and delete items). The list uses square brackets to accommodate all items.
2. Tuple → languages = (“CSS”, “HTML”, “Java”)
Tuple is declare by using round bracket, it is ordered, allow duplicate values, unchangeable(update and delete items not allowed). The Tuple uses round brackets to accommodate all items.
Example
tupleOne = ("one", "two", "three");for tup in tupleOne:
print(tup)OUTPUT:one
two
three
Set Variable
languages = {“CSS”, “HTML”, “Java”}
Set is defined by curly braces, it is unordered, duplicate values are not allowed, unchangeable(update and delete items not allowed). The Set uses curly braces to accommodate all items.
Example
setOne = {"one", "two", "three"};for one in setOne:
print(one)OUTPUT:two
three
one
Map Variable
Map variable is used to store value in key value pair.
Dictionary
A dictionary is one of the types of collections used to store data in key and value pairs.
A dictionary can have int, string, boolean and list as data types.
The dictionary is ordered, changeable, and does not allow duplicate items.
The dictionary item with a duplicate key will always get overridden by the last item.
Example
dictionaryOne = {
"name" : "test",
"id" : "1"
}for key, value in dictionaryOne.items():
print(key, value);OUTPUT:
name test
id 1
Assignment
Assigning a value to a variable called as assignment. Below is the example to assign same ale to multiple variables and unique values to different variables at the same time.

OUTPUT:

Class and Instance Variables
Class variables are those that can be accessed by all instances of a class.
Instance variables are those that can be uniquely accessed by instances of that class.
In the below example, feature is a class variable, and its value will remain the same for c1 and c2 class instances. On the other hand, carType is an instance variable, and its value will depend on the class instance.
e.g. -> cl has carType as Sedan and c2 has carType as Suv
Example

OUTPUT:
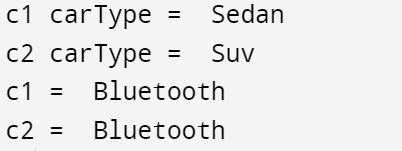
Casting
Casting is all about converting one types of data into another.
If we want to change the data type of any variable, it can be done using the below technique.
Example
In below example, At line 2, 55 is int (on integer), cast it into a string with str at line 3
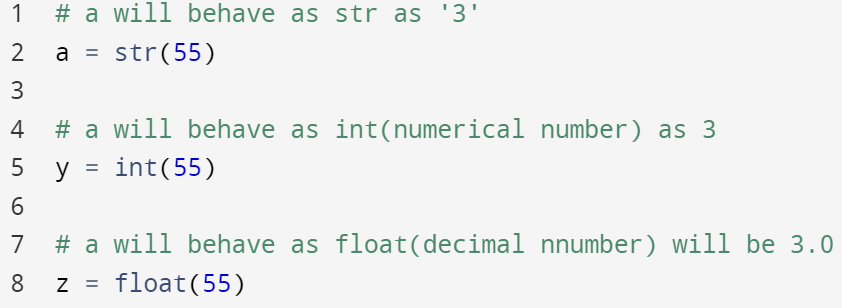
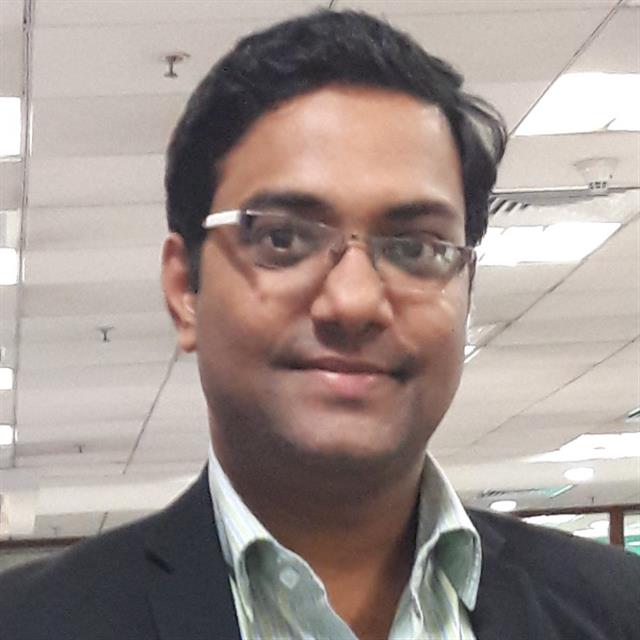
Imran Khan, Adobe Community Advisor, AEM certified developer and Java Geek, is an experienced AEM developer with over 11 years of expertise in designing and implementing robust web applications. He leverages Adobe Experience Manager, Analytics, and Target to create dynamic digital experiences. Imran possesses extensive expertise in J2EE, Sightly, Struts 2.0, Spring, Hibernate, JPA, React, HTML, jQuery, and JavaScript.