Python Exception Handling
Exception handling allows us to handle any exception which occurs at runtime and stop to break the normal flow of a program.
Syntax:
def printName(self, num):
try:
# block of code if exception occurs.
except:
# block of code to handle exception
finally:
# block of code always get call if exception occurs or not
Example:
There are multiple scenarios where developer can get an exception. Below is the best example number divide by zero will given ZeroDivisionError exception.

OUTPUT:

The above example throws an exception at line number 3. The code will not get executed on or after line number 4 as an exception got thrown at line number 3. code threw a ZeroDivisionError exception as 2 was divided by 0 on line 3.
Exception Handling:
Exceptions can be catch by try and except block. The try will contain a block of code which can throw an exception, and the except is to handle the exception gracefully.
Whenever exception occurs, it stops the complete code execution and comes out of the code.
Exception handling will help us to avoid the stoppage of code execution. The below code will execute line number 9 in the case of an exception arising, as it is handled with try and except.

OUTPUT:

finally
Finally block is used to execute for closing an open connections.
Note:
- finally will always get call either exception is thrown or not.
- finally block can be used either with catch or finally.
- finally block can be used with or without catch block.

Example:
In the below example finally will called just after exception is thrown.

OUTPUT:

Raise an Exception:
Custom Exception is all about creating or raising a user defined exception.
Syntax:
In the below example finally will called just after exception is thrown.
Example
In below example code is throwing an exception using raise keyword if variable is not an integer.

OUTPUT:

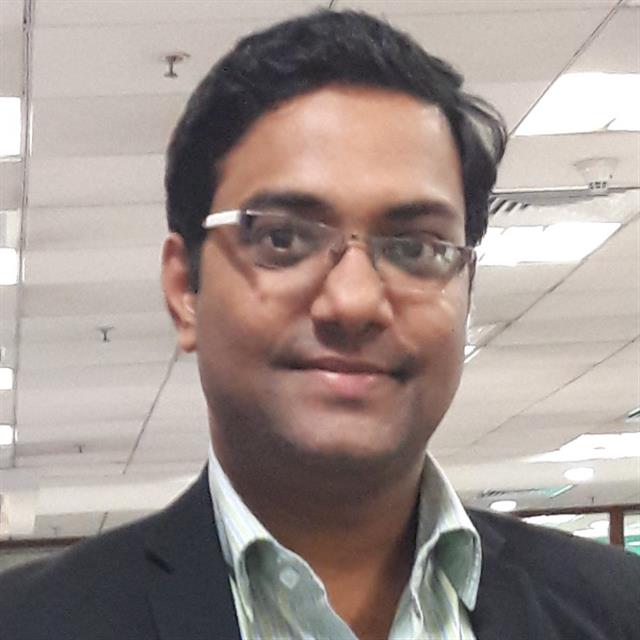
Imran Khan, Adobe Community Advisor, AEM certified developer and Java Geek, is an experienced AEM developer with over 11 years of expertise in designing and implementing robust web applications. He leverages Adobe Experience Manager, Analytics, and Target to create dynamic digital experiences. Imran possesses extensive expertise in J2EE, Sightly, Struts 2.0, Spring, Hibernate, JPA, React, HTML, jQuery, and JavaScript.