Break, Continue and Pass
The break, continue, and pass keywords are used to break, jump, and pass through the loop respectively.
Lets try to understand each and every topic in detail.
Break:
Break keyword terminates the loop as soon as it executes. The break statement will break for, while loop and switch statements.
languages = ["HTML", "CSS", "Java", "Javascript"];
for language in languages:
if language == "Java":
break; print(language)
OUTPUT:
HTML
CSS
Note:
In the preceding example, the loop begins to run and is terminated when the language value is changed to “Java”. After executing the break statement, the loop will not execute any statements inside the loop and come out of it.
Continue:
Continue keyword will allow loop to jump to the next iteration. The continue statement will be applicable for while and for loop.
languages = ["HTML", "CSS", "Java", "Javascript"];for language in languages:
if language == "Java":
continue;
print(language);
OUTPUT:
HTML
CSS
Javascript
Note:
In the above example, the loop starts and prints the value from the languages list. When the language value becomes equal to “java”, the loop gets jumped to the next iteration and doesn’t print or execute any of the statements from the current loop.
Difference between Break and Continue

Pass:
A pass keyword will allow a loop to run an empty statement and pass through. This pass is used to ensure that a block of code is not left empty. This helps us to add a block of code in the future for one of the empty conditions. Leaving a block of code empty under one of the conditions may give a compilation or code quality error by one of the code quality tools.
We can have class with not content and pass helps us to create an empty class. putting pass in an empty class helps us to avoid getting an error on run time.
languages = ["HTML", "CSS", "Java", "Javascript"];for language in languages:
if language == "Java":
pass; # do nothing, just pass through when language is Java.
else:
print(language)
OUTPUT:
HTML
CSS
Javascript
Without pass keyword:
declaring an empty class is giving an error.
class a:OUTPUT:
File “<string>”, line 1
class a:
^
SyntaxError: unexpected EOF while parsing
With pass keyword:
Declaring an empty class with pass keyword is not giving an error as it is not completely empty and having a pass keyword which does nothing.
class a:
pass
OUTPUT:
NOTE: This exactly happens with functions, as declaring an empty function without definition is not allowed. We can also use the pass keyword to avoid runtime errors.
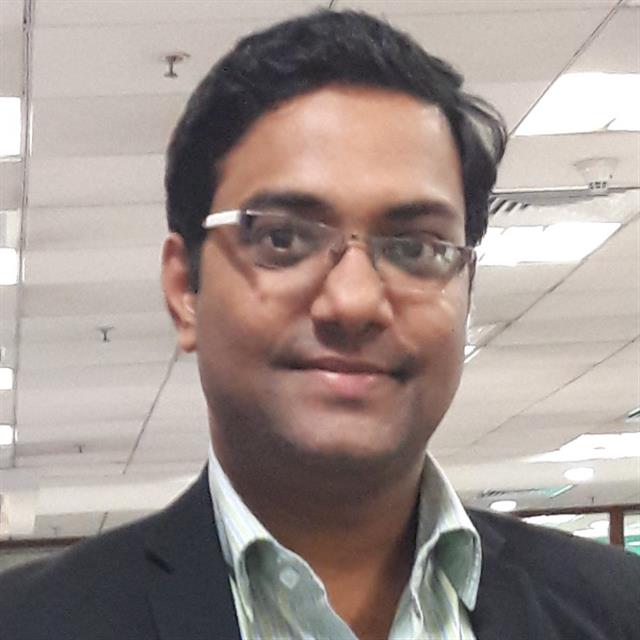
Imran Khan, Adobe Community Advisor, AEM certified developer and Java Geek, is an experienced AEM developer with over 11 years of expertise in designing and implementing robust web applications. He leverages Adobe Experience Manager, Analytics, and Target to create dynamic digital experiences. Imran possesses extensive expertise in J2EE, Sightly, Struts 2.0, Spring, Hibernate, JPA, React, HTML, jQuery, and JavaScript.