Data Structure
This is a complete guide to understanding Python data structures with methods and examples.
This post may be a little bit long. But, it will provide end-to-end complete knowledge of Python data structures, including similarities and differences.
Below are the four data structure defined by Python to store data.
- List → languages = [“CSS”, “HTML”, “Java”]
List is declare by using square bracket, it is ordered, allow duplicate values, changeable(allow update and delete items). The list uses square brackets to accommodate all items.
- Tuple → languages = (“CSS”, “HTML”, “Java”)
Tuple is declare by using round bracket, it is ordered, allow duplicate values, unchangeable(update and delete items not allowed). The Tuple uses round brackets to accommodate all items.
2. Set → languages = {“CSS”, “HTML”, “Java”}
Set is defined by curly braces, it is unordered, duplicate values are not allowed, unchangeable(update and delete items not allowed). The Set uses curly braces to accommodate all items.
3. Dictionary →
dictionaryOne = {
“name” : “test”,
“id” : “123”
}
Dictionary is ordered, duplicate items are not allowed, unchangeable(update and delete items not allowed). The dictionary in similar to JSON object we can

Let’s discuss data structure in detail:
List
Lists store multiple values in a single variable. In the same list variable, list items’ values can be of the same or different type. Each list item can be defined within double quotes separated by commas. The list allows ordered, duplicate, and changeable values.
Example:
list1 = [“HTML”, “CSS”, “Java”];
list2 = [1, 2, 3, 4, 5, 6];
List items can be access using index, it always starts from 0.
e.g. →
Defining a list listOne with four list items HTML, CSS, JavaScript and Java as show below:
listOne = [“HTML”, “CSS”, “JavaScript”, “Java”];
listOne[0] → HTML,
listOne[1] → CSS,
listOne[3] → Java
There are some other methods provided by Python to access list elements:
listOne[0:3] → [‘HTML’, ‘CSS’]
Note: Point to be noted, in above example first item as 0 represents the index number and second item as 3 is number of items.

Example: Below example is all about access, update and delete list items.
listOne = ["HTML", "CSS", "JavaScript", "Java"];
# Accssing single list Item from list
# Accessing multiple list items from
# 0 index and number of items as 3
print(listOne[0:3])
# Updating an element at index in list
listOne[1] = "CSS3";
# Print list items
print(listOne);
#Delete list time at index 2
del listOne[2];
# Print list items
print(listOne);
OUTPUT:
['HTML', 'CSS', 'JavaScript']
['HTML', 'CSS3', 'JavaScript', 'Java']
['HTML', 'CSS3', 'Java']
Below example to sort and create copy of list.
listOne = ["HTML", "CSS", "JavaScript", "Java"];
// sort the list, by default ascending
listOne.sort()
print(listOne);
// sort the list in descending
listOne.sort(reverse = True)
print(listOne);
// Create list copy
listTwo = listOne.copy();
print(listTwo);
OUTPUT:
['CSS', 'HTML', 'Java', 'JavaScript']
['JavaScript', 'Java', 'HTML', 'CSS']
['JavaScript', 'Java', 'HTML', 'CSS']
Tuple
Tuple is also used to store multiple values in a single variable. In the same tuple variable, items’ values can be of the same or different type. Each Tuple item can be defined within double quote separated by a comma. A Tuple allows ordered, duplicated, and unchangeable values.
Example:
tuple1 = (“HTML”, “CSS”, “Java”);
tuple2 = (1, 2, 3, 4, 5, 6);
Tuple items can be access using index always starts from 0.
e.g. →
Defining a list tupleOne with four items HTML, CSS, JavaScript and Java as show below:
tupleOne = [“HTML”, “CSS”, “JavaScript”, “Java”];
tupleOne[0] → HTML,
tupleOne[1] → CSS,
tupleOne[3] → Java
tupleOne = ("HTML", "CSS", "JavaScript", "Java");
# Accssing single tuple Item from list
print(tupleOne[1]);
# Accessing multiple tuple items from
# 0 index and number of items as 3
print(tupleOne[0:3]);
OUTPUT:
('HTML', 'CSS', 'JavaScript')
('HTML', 'CSS', 'JavaScript', 'Java')
('HTML', 'CSS', 'JavaScript', 'Java')
Below list example is to add, update and check if item exist in list.
listOne = {"HTML", "JavaScript"};
# It will check 'HTML' exist or not
print("HTML" in listOne);
# Add list in Java
listOne.add("Java");
print(listOne);
# Add or updpate CSS in list
listOne.update("CSS");
print(listOne);
# update listOne with listTwo items
listTwo = {"C", "C++"};
listOne.update(listTwo);
print(listOne);
OUTPUT:
True
{'HTML', 'Java', 'JavaScript'}
{'JavaScript', 'C', 'S', 'HTML', 'Java'}
{'C++', 'JavaScript', 'C', 'S', 'HTML', 'Java'}
NOTE: Tuple doesn’t allow assignment(update) and deletion of item.
Set
Set is also used to store multiple values. In the same Set variable, items’ values can be of the same or different type. Each set item can be defined within curly braces by having a double quote separated by a comma. A set allows only unique, non-duplicated, and unchangeable values.
In Set, items are access using index. Set items can only be loop through using loop.
Example:
set1 = {“HTML”, “CSS”, “Java”};
set2 = {1, 2, 3, 4, 5, 6};
Set items can be access using index always starts from 0.
e.g. →
Defining a list setOne with four items HTML, CSS, JavaScript and Java as show below:
setOne = {"HTML", "CSS", "JavaScript", "Java"};
for one in setOne:
print(one);OUTPUT:
HTML
JavaScript
CSS
Java
Set Operations → add, update, clear, delete item and list.
Please read below code snippet with comment will help to get better code understanding.
# Create a set with one, two and three itmes
setOne = {"one", "two", "three"};
# Add 4th item four as a value
setOne.add("four");
print(setOne);
# Update the setOne with setTwo items
setTwo = {"five"};
setOne.update(setTwo);
print(setOne);
# Update set(setOne) with list(listOne)items.
listOne = ["six", "seven"]
setOne.update(listOne);
print(setOne);
# Remove an item from setOne
setOne.remove("two");
print(setOne);
# discard an item from setOne
setOne.discard("three");
print(setOne);
# clear the setOne Set, remove or delete all its items and print empy set.
setOne.clear();
print(setOne);
# It will delete Set including its items
# It will give an erro while printing the same.
del setOne;
print(setOne);
OUTPUT:
{'four', 'one', 'two', 'three'}
{'four', 'one', 'five', 'two', 'three'}
{'four', 'seven', 'one', 'six', 'five', 'two', 'three'}
{'four', 'seven', 'one', 'six', 'five', 'three'}
{'four', 'seven', 'one', 'six', 'five'}
set()
Traceback (most recent call last):
File "<string>", line 33, in <module>
NameError: name 'setOne' is not defined
In the above example, the remove() method will give an error in case the item being removed doesn’t exist in the set. In the case of discard, however, no errors will be thrown.
We can also use the pop() function to remove an item. We never know what will be deleted from a set because it is an unordered list.
Dictionary
A dictionary is one of the types of collections used to store data in key and value pairs.
A dictionary can have int, string, boolean and list as data types.
The dictionary is ordered, changeable, and does not allow duplicate items.
The dictionary item with a duplicate key will always get overridden by the last item.
dictionaryOne = {
"name" : "test",
"id" : "1"
}for key, value in dictionaryOne.items():
print(key, value);OUTPUT:
name test
id 1
Below is the example to access value from dictionary using key:
dictionaryOne = {
"name" : "test",
"id" : "1"
}
print(dictionaryOne.get("name"));OUTPUT:
test
Methods in Dictionary
Below Dictionary methods is all about access, add, update and exist Dictionary item.
Please read the below code snippet with comments. This will help you get a better code understanding.
dictionaryOne = {
"name" : "test",
"id" : "1"
}
# get all keys from dictionary
print(dictionaryOne.keys());
# get all values from dictionary
print(dictionaryOne.values());
# get all items as key and value pair from dictionary
print(dictionaryOne.items());
#add or update an item in Disctionary
dictionaryOne.update({"age": "32"});
print(dictionaryOne.items());
#add or update an item in Disctionary
dictionaryOne["age"] = "30";
print(dictionaryOne.items());
# Check if particular key exist or not in Dictionary
if "name" in dictionaryOne:
print("name exist in Dictionary!!");
OUTPUT:
dict_keys(['name', 'id'])
dict_values(['test', '1'])
dict_items([('name', 'test'), ('id', '1')])
dict_items([('name', 'test'), ('id', '1'), ('age', '32')])
dict_items([('name', 'test'), ('id', '1'), ('age', '30')])
name exist in Dictionary!!
Below is an example to remove item or all items from Dictionary.
dictionaryOne = {
"name" : "test",
"id" : "1",
"age" : "30",
"address" : "address 1"
}
# pop will remove item using key
print(dictionaryOne.pop("name"));
# remove itmem which inserted in last
print(dictionaryOne.popitem());
# del will remove item using key
del dictionaryOne["age"]
print(dictionaryOne);
# empty Dictionary
print(dictionaryOne.clear());OUTPUT:
test
('address', 'address 1')
{'id': '1'}
None
Copy Dictionary
The following code will help us to create a complete copy of items from the existing Dictionary to the new one.
dictionaryOne = {
"name" : "test",
"id" : "1",
"age" : "30",
"address" : "address 1"
}
print(dictionaryOne);
# This is not a copy
# just a reference update to dictionaryOne
dictionarytwo = dictionaryOne;
print(dictionarytwo);
# Making a copy() of Dictionary
dictionaryThree = dictionaryOne.copy();
print(dictionaryThree);
OUTPUT:
{'name': 'test', 'id': '1', 'age': '30', 'address': 'address 1'}
{'name': 'test', 'id': '1', 'age': '30', 'address': 'address 1'}
{'name': 'test', 'id': '1', 'age': '30', 'address': 'address 1'}
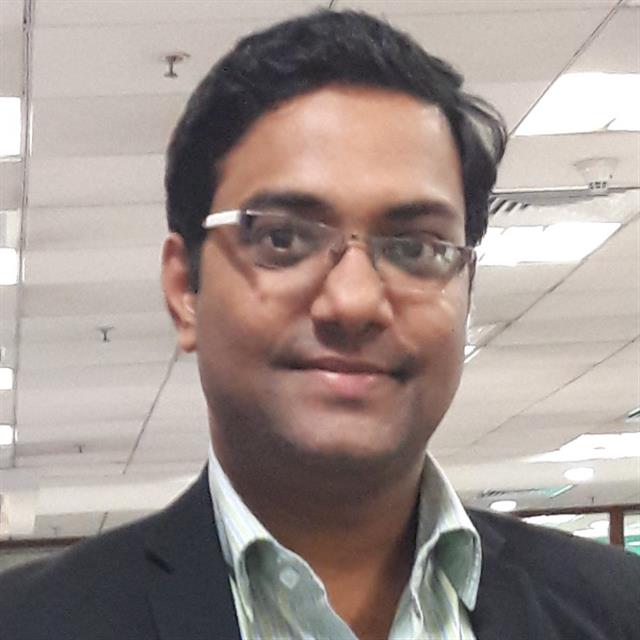
Imran Khan, Adobe Community Advisor, AEM certified developer and Java Geek, is an experienced AEM developer with over 11 years of expertise in designing and implementing robust web applications. He leverages Adobe Experience Manager, Analytics, and Target to create dynamic digital experiences. Imran possesses extensive expertise in J2EE, Sightly, Struts 2.0, Spring, Hibernate, JPA, React, HTML, jQuery, and JavaScript.