Spring Boot Profiles
Spring Boot profiles allow us to configure variables or values based on different environments such as default, DEV, STAGE, and PROD.
In short, it enable us to define environment-specific variable values without making any code change. For example, if our MySQL server has different ports, usernames, and passwords for DEV, QA, and STAGE environments, we can manage them using profiles.
Spring boot application by default creates a file at the time of project creation named as application.properties file. This file contains both system define and custom properties.
Flow link to read more about application properties and how it works.
Below is an example to define property inside application.properties file.
Here, # denotes for writing a comment.
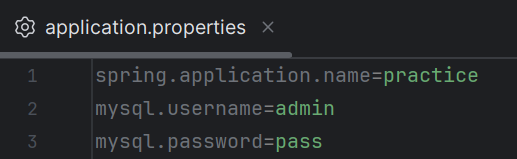
Create EmployeeService component class to read values from application properties with the help of @Value annotation as show below from application.properties by default:
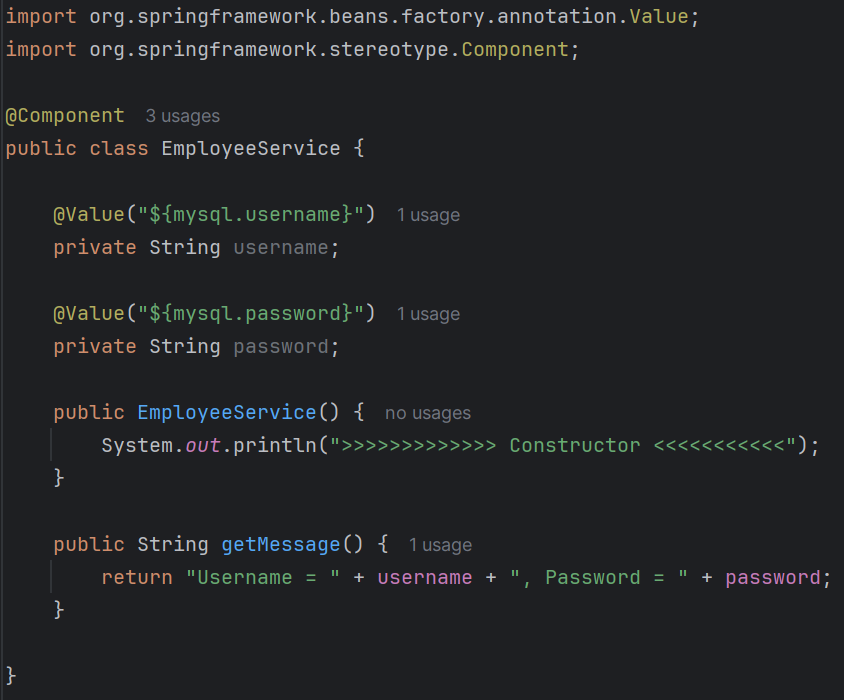
Below is the output post running spring boot application:
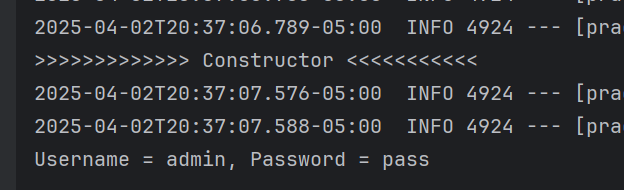
Similar to application.properties, we have environment specific properties file such as application-dev.properties, application-qa.properties and
application-prod.properties for DEV, QA and PROD environments respectively as shown below:
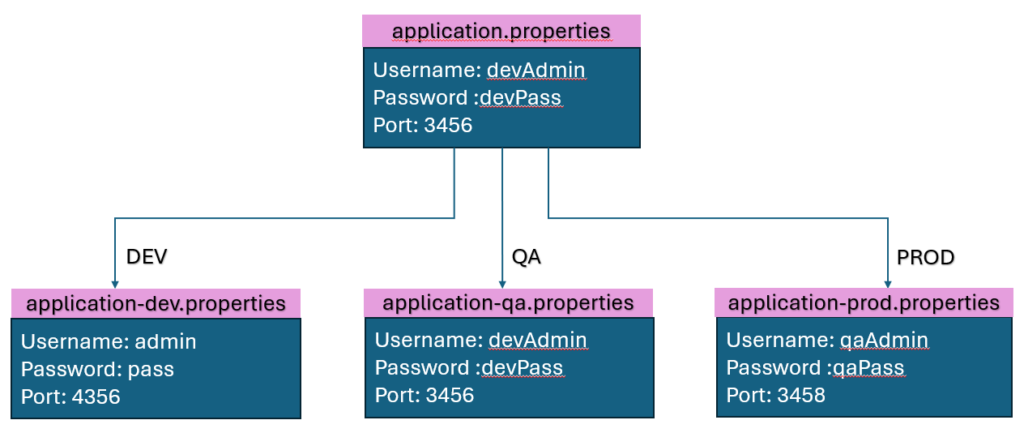
Note: Application will fall back to application.properties if required profile not found or created.
Below is an example of application-qa.properties having username and password properties:
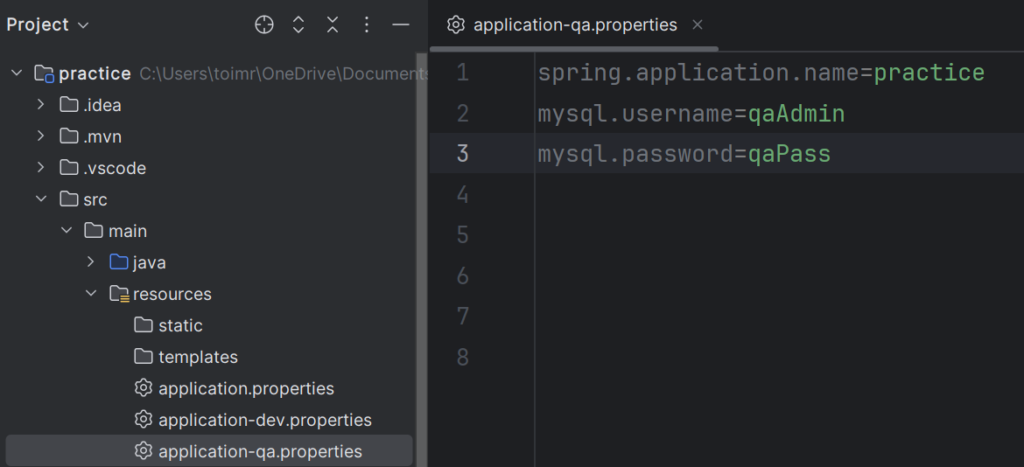
Declare below highlighted property spring.profiles.active=qa as part of application.properties file to consider or enable properties from given environment.
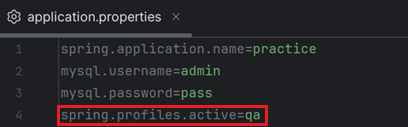
Now, running the application will give below output reading properties from application-qa.properties file:
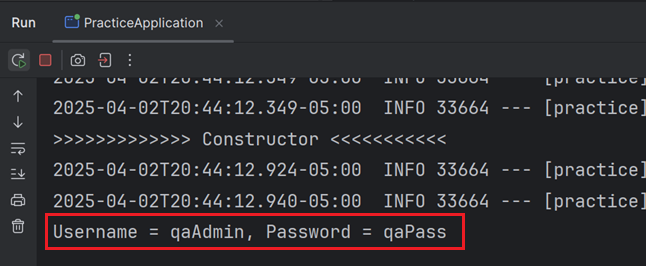
There are some other below ways to set environment:
Config Environment at the time of Application start as part of command
There is one more way where we can update environment value at the time of application start as shown below.
Running below command at the time of application startup will automatically start picking up properties from QA application properties file.
mvn spring-boot:run -Dspring-boot.run.profiles=dev
Note: Code will start ignoring spring.profiles.active=qa defined in application.properties file.
Define Profile ID in pom.xml
In pom.xml, we can create multiple profiles as shown below for DEV, QA and STAGE:
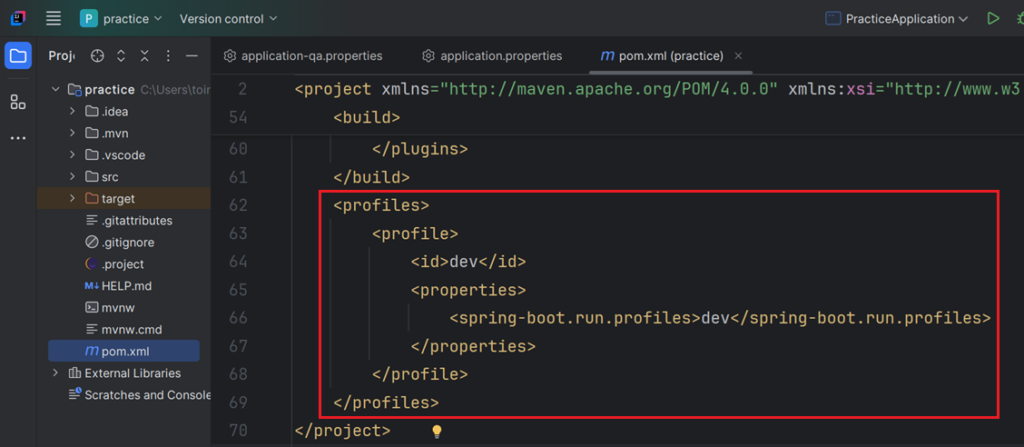
Follow below syntax to run above command:
mvn spring-boot:run -P<profile_id>
Run below command to start application and this time it will conside DEV profile:
mvn spring-boot:run -Pdev
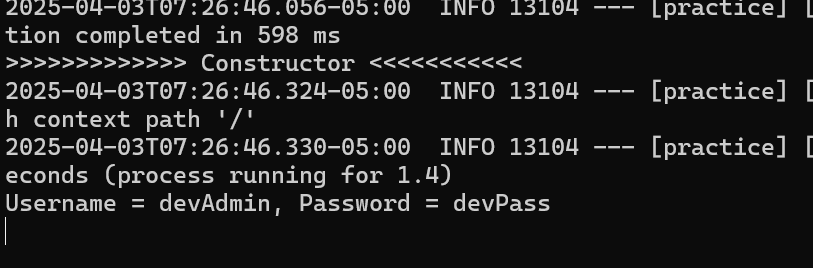
@Profile Annotation
Use @Profile annotation allow us to load bean depending on the environment.
For example, below EmployeeComponent will only get register / applicable only for DEV based on declared profile.
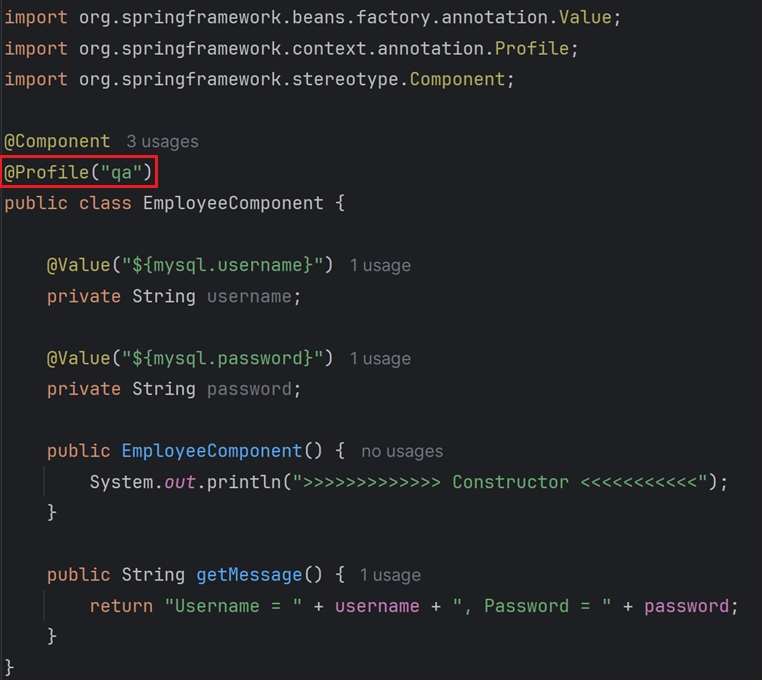
Run below command considering dev as profile:
mvn spring-boot:run -Pdev
It will give below inline error as we marked EmployeeComponent only applicable for qa:
Exception in thread “main” org.springframework.beans.factory.NoSuchBeanDefinitionException: No qualifying bean of type ‘com.example.demo.beans.EmployeeComponent’ available

Multiple Profiles:
Note: In application.properties, we can declare multiple profiles as comma separated.
spring.profiles.active=qa,dev
- Both application-dev.properties and application-qa.properties will be loading.
- If a property exists in multiple profiles, the last profile in the list takes precedence.
Merge Profiles
If we are loading dev and qa profile together using below command:
spring.profiles.active=qa,dev
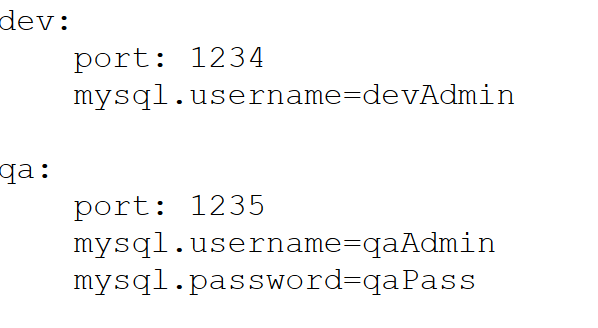
Here, last dev profile will take the precedence.
Code will consider port and username from dev as it positioned in last.
For password, it will fallback to QA as it is not defined in DEV.
Final Output:
port: 1234
mysql.username=devAdmin
mysql.password=qaPass
Set Default Profile Explicitly
We can default profile explicitly. Code will consider default profile if required profile not found:
spring.profiles.default=dev
Access Profiles in Code
We can access both active and default profiles in Java source code declared in application.properties file.
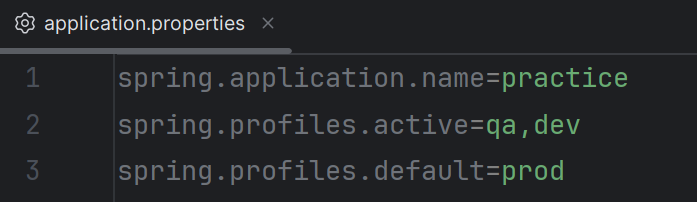
Using context we can get Environment and using it we can access active and default profiles:
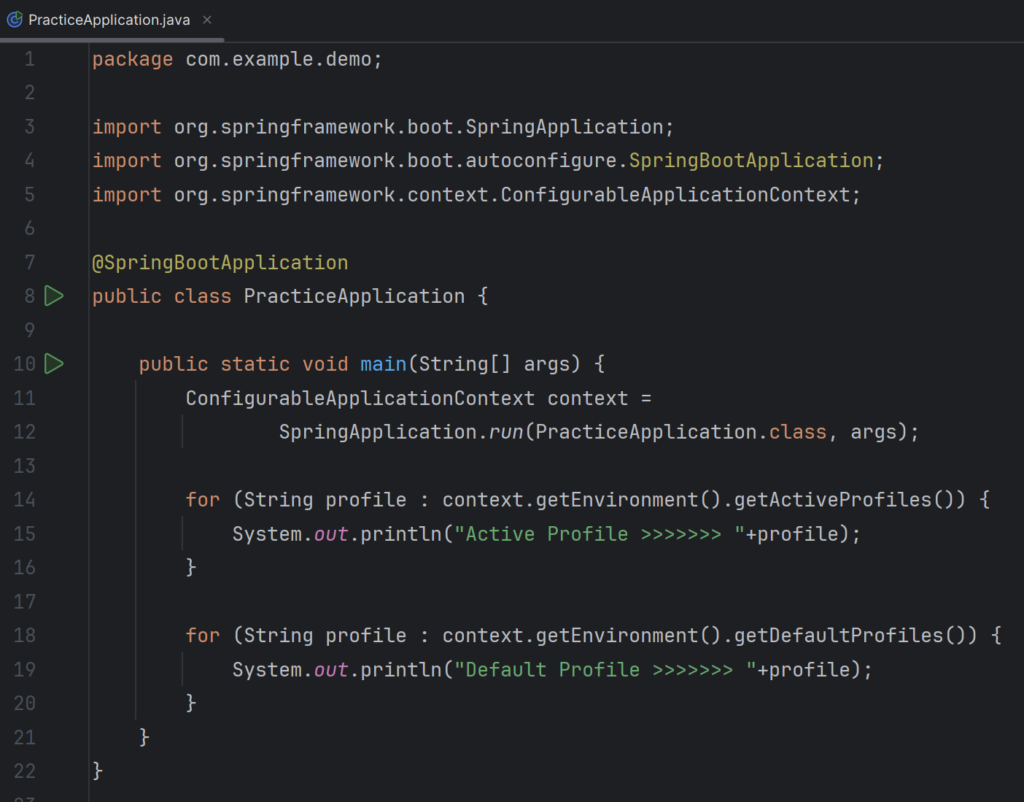
OUTPUT:
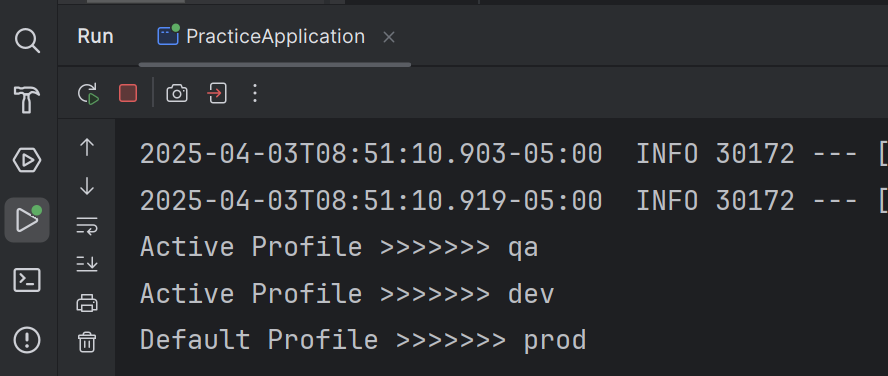
Learn more about Spring Boot
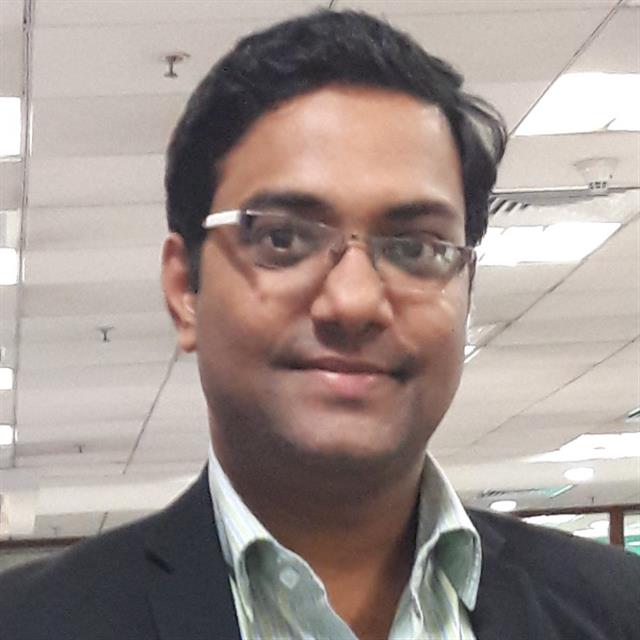
Imran Khan, Adobe Community Advisor, certified AEM developer and Java Geek, is an experienced AEM developer with over 12 years of expertise in designing and implementing robust web applications. He leverages Adobe Experience Manager, Analytics, and Target to create dynamic digital experiences. Imran possesses extensive expertise in J2EE, Sightly, Struts 2.0, Spring, Hibernate, JPA, React, HTML, jQuery, and JavaScript.