State
State belongs to a component and used to store data or object. Change in state object will always re-render the view. State denotes the current state of component.
As a best and recommended approach to use setState() method to update data or object. Updating state object or data using setState() method helps us to re-render view without any delay.
Follow below steps to work with component state:
- Create react project using npx create-react-app practice-app command. practice-app is the custom project name.

2. Paste below code inside App.js file.
import React from 'react';
import logo from './logo.svg';
import './App.css';
class App extends React.Component {
constructor(props) {
super(props);
this.state = {
id: 1,
name: "John"
};
}
render() {
return (
<div>
<h2>Id: {this.state.id}</h2>
<h2>Name: {this.state.name}</h2>
</div>
);
}
}
export default App;
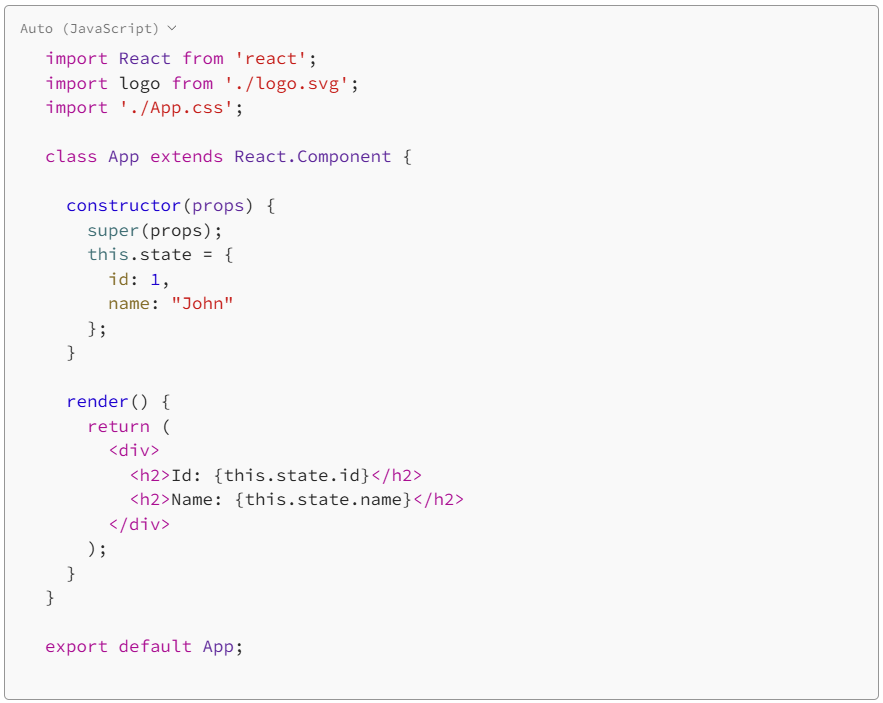
3. Run React application using npm start command as shown below:

4. Hit url to load ReactJS application and check output as shown below:
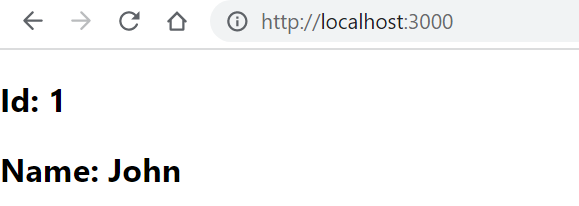
setState() Method Example
Below is an example to see the change in state and re-render the view.
Update App.js file with below content. We have added changeName() method to update state of name property.
Call changeName() method to update state of name on click of button using onClick event.
import React from 'react';
import logo from './logo.svg';
import './App.css';
class App extends React.Component {
constructor(props) {
super(props);
this.state = {
id: 1,
name: "John"
};
}
changeName = () => {
this.setState({name: "Carter"});
}
render() {
return (
<div>
<h2>Id: {this.state.id}</h2>
<h2>Name: {this.state.name}</h2>
<button
type="button"
onClick={this.changeName}
>Update Name</button>
</div>
);
}
}
export default App;
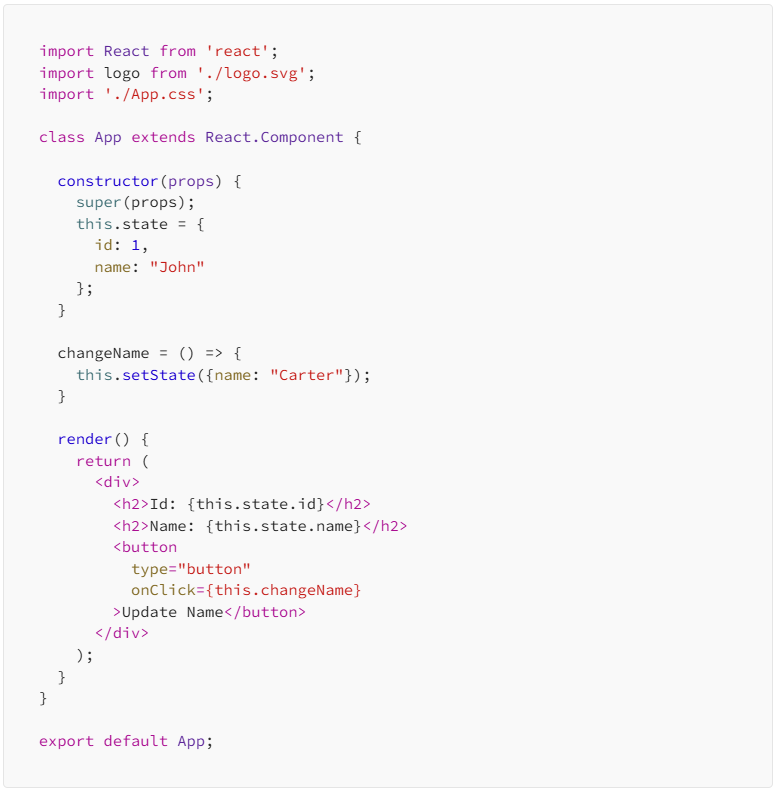
3. Run React application using npm start command as shown below:

4. Hit url to load ReactJS application and check output as shown below on page load:
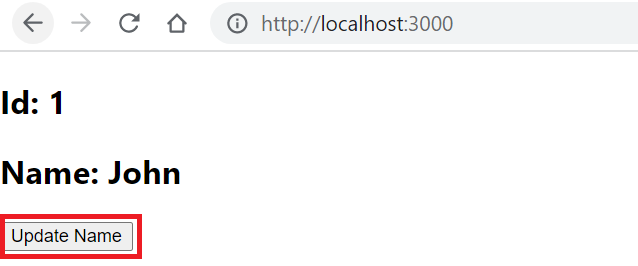
5. Clicking on Update Name button will update name from John to Carter as shown below.
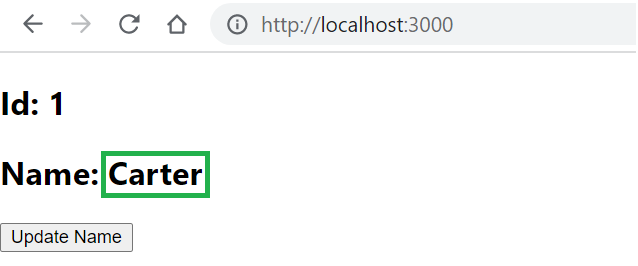
State Vs Props
Below is the difference with state and props:

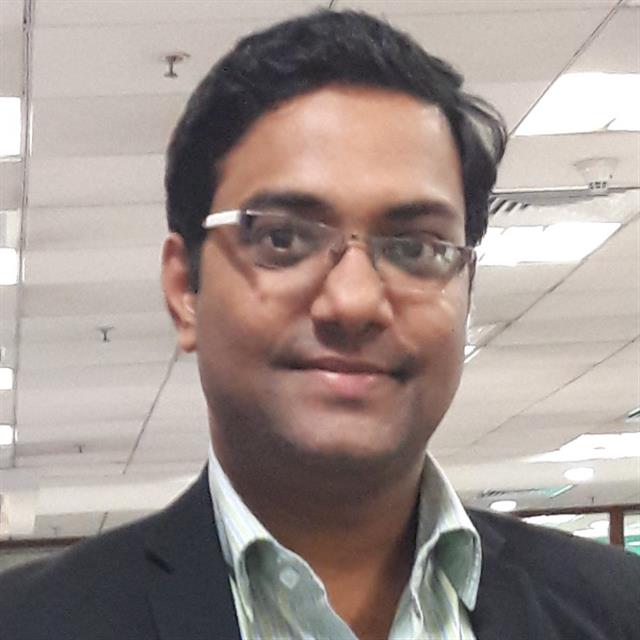
Imran Khan, Adobe Community Advisor, certified AEM developer and Java Geek, is an experienced AEM developer with over 12 years of expertise in designing and implementing robust web applications. He leverages Adobe Experience Manager, Analytics, and Target to create dynamic digital experiences. Imran possesses extensive expertise in J2EE, Sightly, Struts 2.0, Spring, Hibernate, JPA, React, HTML, jQuery, and JavaScript.