React Forms
React allow us to create Forms. Its complex to work with forms in React as it require to integrate with hook.
Example
Below is the custom sample code to create React Forms without using any Hook:
- Create user object having name and age as part of useState hook. Map field with <input/> element created as part of these fields.
- Create custom handleInputChange method to collect value on change of any input field.
- Create custom handleSubmit function and print user object with all values collected as part of handleInputChange.
import React, { useState } from "react";
export default function App() {
const [user, setUser] = useState({
name: "",
age: ""
});
const handleInputChange = (event) => {
const { name, value } = event.target;
setUser((prevProps) => ({
...prevProps,
[name]: value
}));
};
const handleSubmit = (event) => {
event.preventDefault();
console.log(user);
};
return (
<div className="App">
<form onSubmit={handleSubmit}>
<div className="form-control">
<label>Name</label>
<input
type="text"
name="name"
value={user.name}
onChange={handleInputChange}
/>
</div>
<div className="form-control">
<label>Age</label>
<input
type="text"
name="age"
value={user.age}
onChange={handleInputChange}
/>
</div>
<div className="form-control">
<label></label>
<button type="submit">Submit</button>
</div>
</form>
</div>
);
}
OUTPUT
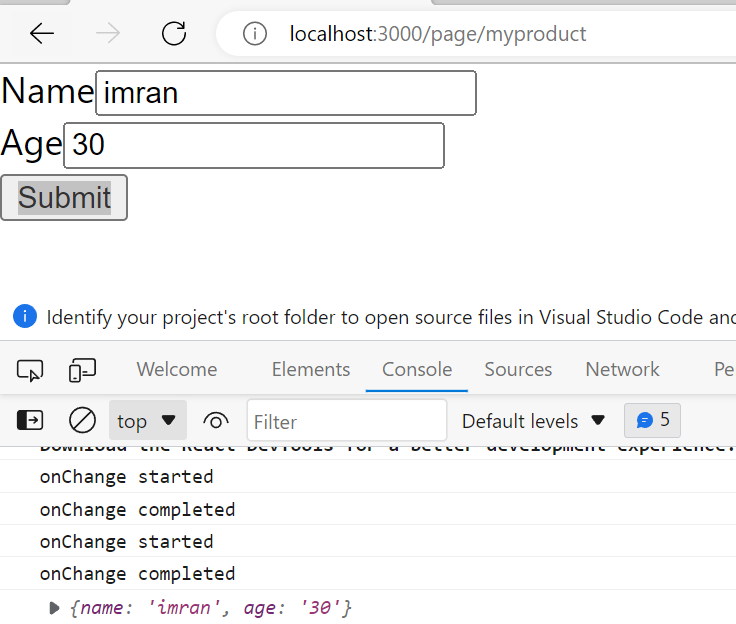
In the above form, it is quite difficult to write boilerplate or custom code to collect values from input fields. We can use react-hook-form which OOTB provides us the capability to collect value in the object.
react-hook-form
Use below command to install react-hook-form
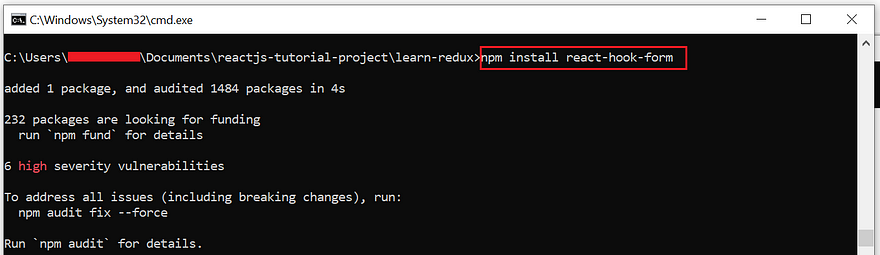
Example:
In below code snippet, there is no need to write onChange event and useState hook as we are using react-hook-form
import React from "react";
import { useForm } from "react-hook-form";
export default function App() {
const {
register,
handleSubmit,
} = useForm();
const onSubmit = (data) => {
console.log(data);
};
return (
<div className="App">
<form onSubmit={handleSubmit(onSubmit)}>
<input type="text" name="name" {...register("email")}/>
<input type="age" name="age" {...register("age")}/>
<button type="submit">Submit</button>
</form>
</div>
);
}
OUTPUT
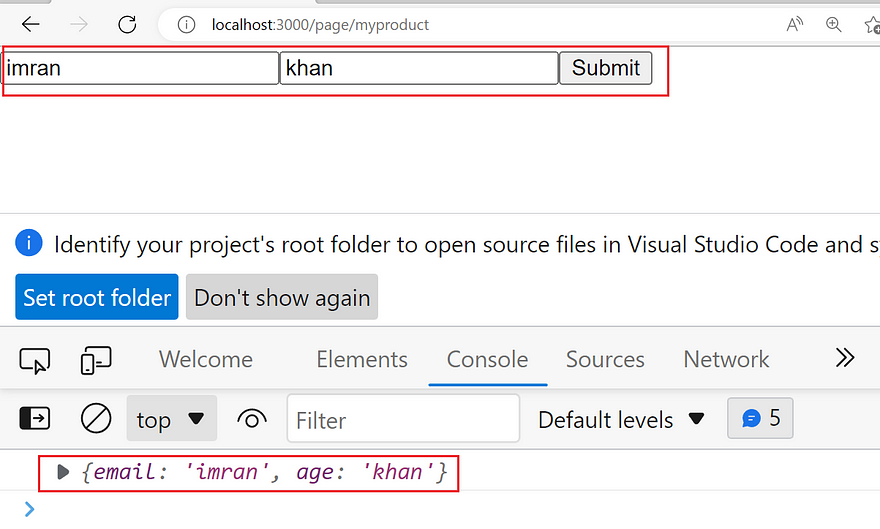
Form Validation:
Below is the form validation example to check required and name must be having only characters.
import React from "react";
import { useForm } from "react-hook-form";
export default function App() {
const {
register,
handleSubmit,
formState: { errors }
} = useForm();
const onSubmit = (data) => {
console.log(data);
};
return (
<div className="App">
<form onSubmit={handleSubmit(onSubmit)}>
<input type="text" name="name" {...register("name",{required: true, pattern: /^[a-zA-Z]$/})}/>
{errors.name && errors.name.type === "required" && (
<p className="errorMsg">Name is required.</p>
)}
{errors.name && errors.name.type === "pattern" && (
<p className="errorMsg">Not valid Name.</p>
)}
<input type="text" name="age" {...register("age")}/>
<button type="submit">Submit</button>
</form>
</div>
);
}
OUTPUT
Below is require field validation example:
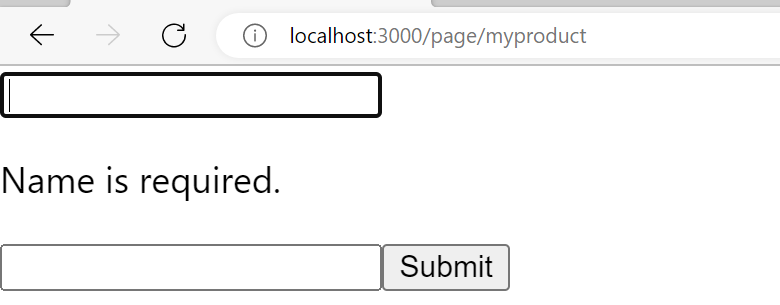
Below is not valid name example:
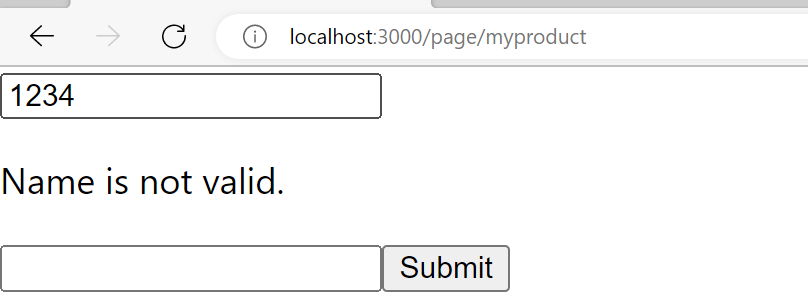
Initialize form value on page load
Below is an example to load form with values using useForm defaultValues object.
import React from "react";
import { useForm } from "react-hook-form";
export default function App() {
const {
register,
handleSubmit,
formState: { errors }
} = useForm({
defaultValues: {
name: "imran",
age: 23
}
});
return (
<div className="App">
<form>
<input type="text" name="name" {...register("name")}/>
<input type="text" name="age" {...register("age")}/>
<button type="submit">Submit</button>
</form>
</div>
);
}
OUTPUT

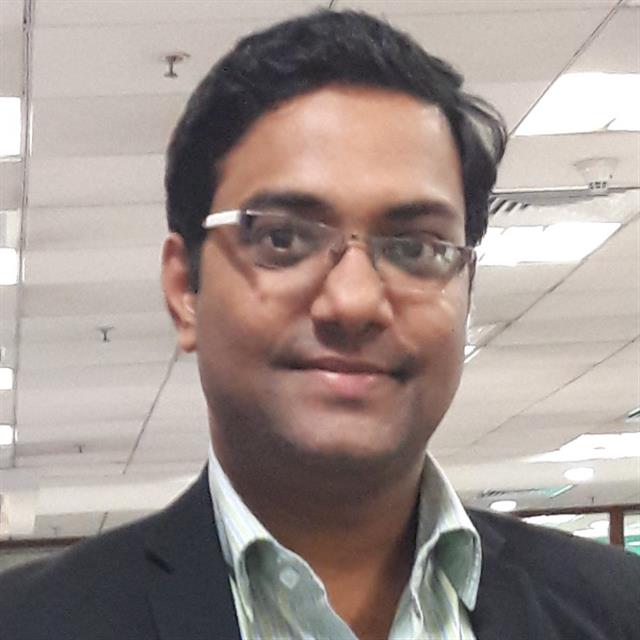
Imran Khan, Adobe Community Advisor, AEM certified developer and Java Geek, is an experienced AEM developer with over 11 years of expertise in designing and implementing robust web applications. He leverages Adobe Experience Manager, Analytics, and Target to create dynamic digital experiences. Imran possesses extensive expertise in J2EE, Sightly, Struts 2.0, Spring, Hibernate, JPA, React, HTML, jQuery, and JavaScript.