Data Layer
Data Layer is a JavaScript object contains page data. Data Layer object allow us to pass data from site to Adobe analytics.
Ensure that the site development team takes primary responsibility for ensuring the correct population of data layer objects with accurate values. Review this page with your site development team to align expectations between teams effectively.
Adobe’s recommended data layer specifications is optional. If you already have a data layer, or otherwise choose not to follow Adobe’s specifications, make sure that your organization aligns on what specification to follow.
To access any data layer from the website, open the browser console, navigate to the page’s digitalData or window.dataLayer depending on the implementation, and press enter.
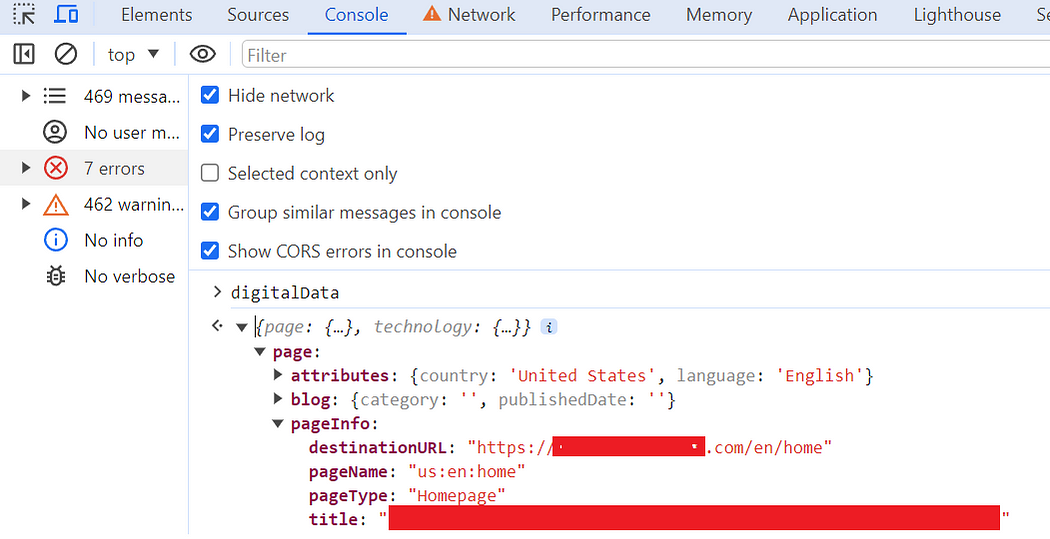
Below is the sample data layer which is having site and current page data. We can have more object within data layer having information around user after login, product data on product details page. This is one of the way to identify most or less viewed product by looking its page insight.
{
"page": {
"pageInfo": {
"pageName": "us:en:home",
"pageType": "Homepage",
"destinationURL": "https://test.com/en/home",
"title": "this is test home page | Home Page"
},
"attributes": {
"country": "United States",
"language": "English"
},
"blog": {
"category": "",
"publishedDate": ""
},
"releaseNotes": {
"updatedDate": "",
"publishedDate": ""
},
"productInfo": {
"name": "shoe",
"id": "1234"
}
}
}
Data Layer Implementation
As previously mentioned, the implementation of the Data Layer will be handled by the Site Developer, and it may differ from site to site and client to client. Below is a sample illustrating how to create the Data Layer.
Below is the sample to create to create Data Layer.
- Create an interface having all required data layer variables such as page name, page title, language, site name, hierarchy, page category, etc.
package com.javadoubts.core.models;
public interface DataLayerModel {
String getPageLanguage();
String getPageName();
String getPageCategory();
String getSiteVersion();
String getSiteName();
String getPageHierarchy();
}
2. Create Sling model implementing above DataLayerModel interface. Implementation is totally depends to clients requirement.
package com.javadoubts.core.models.impl;
import com.day.cq.commons.inherit.HierarchyNodeInheritanceValueMap;
import com.day.cq.commons.inherit.InheritanceValueMap;
import com.day.cq.commons.jcr.JcrConstants;
import com.day.cq.wcm.api.Page;
import com.day.cq.wcm.api.Template;
import com.javadoubts.core.models.DataLayerModel;
import org.apache.commons.lang3.StringUtils;
import org.apache.sling.api.SlingHttpServletRequest;
import org.apache.sling.api.resource.Resource;
import org.apache.sling.models.annotations.DefaultInjectionStrategy;
import org.apache.sling.models.annotations.Model;
import javax.annotation.PostConstruct;
import javax.inject.Inject;
import java.util.Locale;
@Model(adaptables = {SlingHttpServletRequest.class,
Resource.class},
adapters = DataLayerModel.class,
defaultInjectionStrategy = DefaultInjectionStrategy.OPTIONAL)
public class DataLayerModelImpl implements DataLayerModel {
private static final String SITE_NAME = "Practice Site";
private static final int HOME_PAGE_LEVEL = 3;
private static final String HYPHEN_WITH_SPACE = " - ";
/** The current page. */
@Inject
private Page currentPage;
/** The current page language. */
private String pageLanguage;
/** The current name. */
private String pageName;
/** The current page category. */
private String pageCategory;
/** The page hierarchy. */
private String pageHierarchy = "NA";
/** The site name. */
private String siteName;
/** The site version. */
private String siteVersion;
/** The page template. */
private String pageTemplate;
/**
* Gets invoked after completion of all injections.
*
*/
@PostConstruct
protected void init() {
if (null != currentPage) {
InheritanceValueMap ivm = new HierarchyNodeInheritanceValueMap(currentPage.getContentResource());
pageCategory = ivm.getInherited("category", String.class);
if (StringUtils.isNotEmpty(pageCategory)) {
pageName = pageCategory + "|" + currentPage.getTitle();
} else {
pageName = currentPage.getTitle();
}
pageLanguage = currentPage.getAbsoluteParent(3).getTitle();
setPageHierarchy();
Template template = currentPage.getTemplate();
if (null != template) {
pageTemplate = template.getTitle();
}
}
siteName = SITE_NAME;
InheritanceValueMap ivm = new HierarchyNodeInheritanceValueMap(currentPage.getContentResource());
String language = ivm.getInherited(JcrConstants.JCR_LANGUAGE, String.class);
if (StringUtils.isNotEmpty(language)) {
Locale locale = new Locale(language);
siteName = SITE_NAME + HYPHEN_WITH_SPACE + pageLanguage;
}
}
private void setPageHierarchy() {
if (currentPage.getDepth() > HOME_PAGE_LEVEL + 1) {
pageHierarchy = StringUtils.EMPTY;
for (int i = HOME_PAGE_LEVEL; i < currentPage.getDepth(); i++) {
if (i >= HOME_PAGE_LEVEL) {
if(i > HOME_PAGE_LEVEL && i < currentPage.getDepth() &&
currentPage.getDepth() != HOME_PAGE_LEVEL + 1) {
pageHierarchy = pageHierarchy + "-";
}
pageHierarchy = pageHierarchy + currentPage.getAbsoluteParent(i).getTitle();
}
}
}
}
@Override
public String getPageLanguage() {
return pageLanguage;
}
@Override
public String getPageName() {
return pageName;
}
@Override
public String getPageCategory() {
return pageCategory;
}
@Override
public String getSiteVersion() {
return siteVersion;
}
@Override
public String getSiteName() {
return siteName;
}
public String getPageTemplate() {
return pageTemplate;
}
@Override
public String getPageHierarchy() {
return pageHierarchy;
}
}
3. Create below datalayer.html as part of page component so that it will be available for all page components by default.
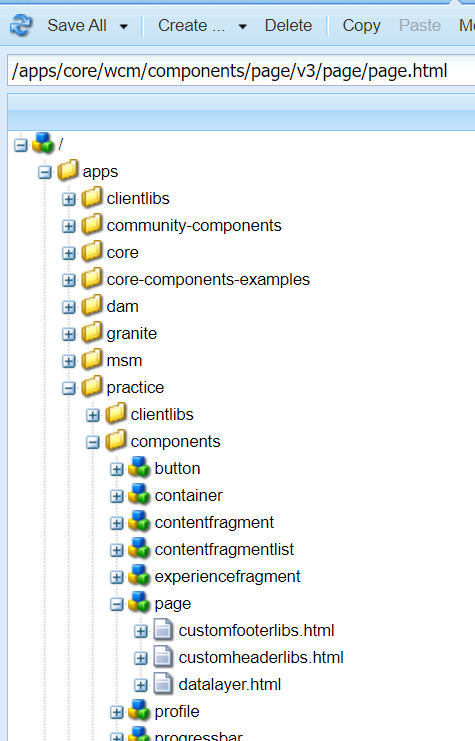
Copy paste below code under datalayer.html file
<sly data-sly-use.dataLayerHelper="${'com.javadoubts.core.models.DataLayerModel'}"/>
<script>
window.dataLayer = window.dataLayer || [];
dataLayer.push({
event:"page_view",
pageinfo: {
page_title:"${dataLayerHelper.pageName @context='text'}",
page_location:window.location.href,
page_category:"${dataLayerHelper.pageCategory @context='text'}",
page_hierarchy:"${dataLayerHelper.pageHierarchy @context='text'}",
page_language:"${dataLayerHelper.pageLanguage @context='text'}",
page_template:"${dataLayerHelper.pageTemplate @context='text'}"
},
siteinfo: {
site_name:"${dataLayerHelper.siteName @context='text'}",
site_version:"${dataLayerHelper.siteVersion @context='text'}"
}
});
</script>
4. Include datalayer.html as part of headerlibs.html or page.html file in the end of the file
<sly data-sly-include="datalayer.html"></sly>
5. Open any page in browser, open console and paste window.dataLayer as shown below:
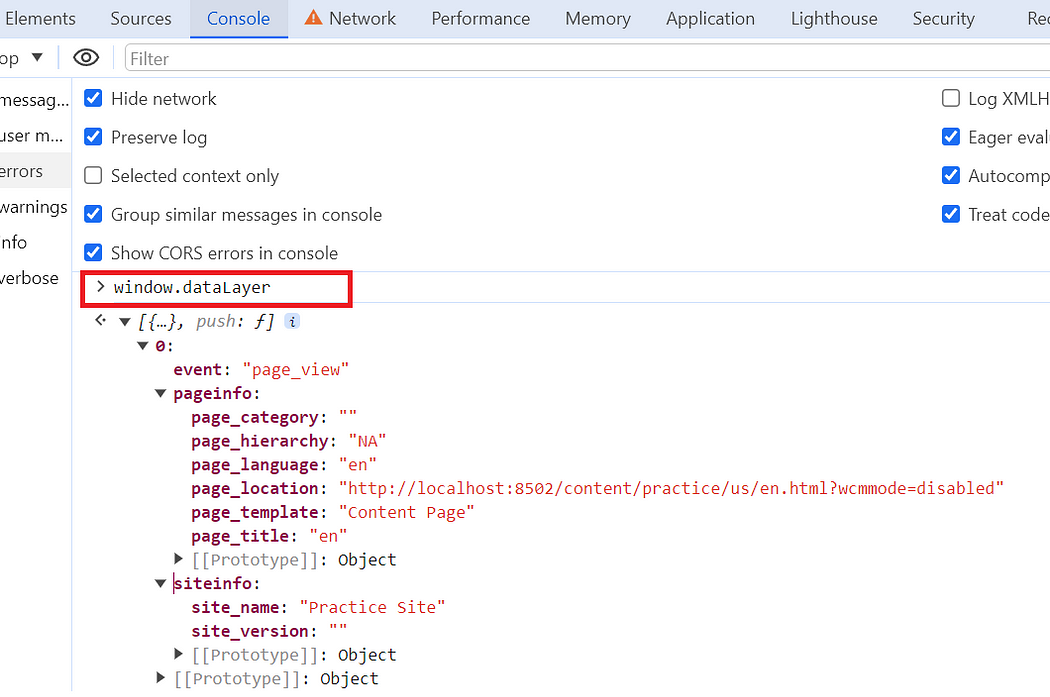
Important Note: Follow link to see overall usage of Data Layer as part of Adobe Analytics.
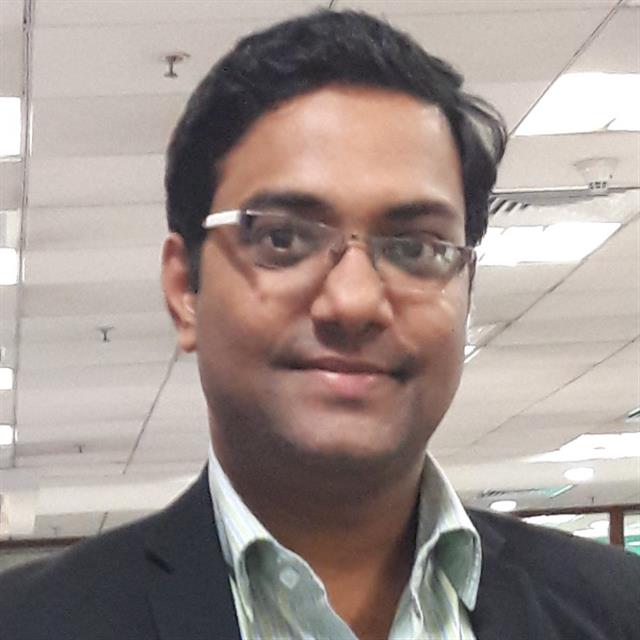
Imran Khan, Adobe Community Advisor, certified AEM developer and Java Geek, is an experienced AEM developer with over 12 years of expertise in designing and implementing robust web applications. He leverages Adobe Experience Manager, Analytics, and Target to create dynamic digital experiences. Imran possesses extensive expertise in J2EE, Sightly, Struts 2.0, Spring, Hibernate, JPA, React, HTML, jQuery, and JavaScript.