React List
This blog will help us to work with React List, loop and render it on the page using ES6 JavaScript map() function.
Follow below steps to create React List component.
- Create react project using npx create-react-app practice-app command. practice-app is the custom project name.

2. Create Main.js file to create Main Class component to play with List.
import React from 'react';
Example:
Below is an example to identify the lenght of he list and print its all items.
import React from "react";
class App extends React.Component {
students = [1, 2, 3, 4];
render() {
return (
<div>
<div>
<div> Number of Students = {this.students.length}</div>
<div>{
this.students.map((student) => <div>{student}</div>)
}
</div>
</div>
</div>
);
}
}
export default App;
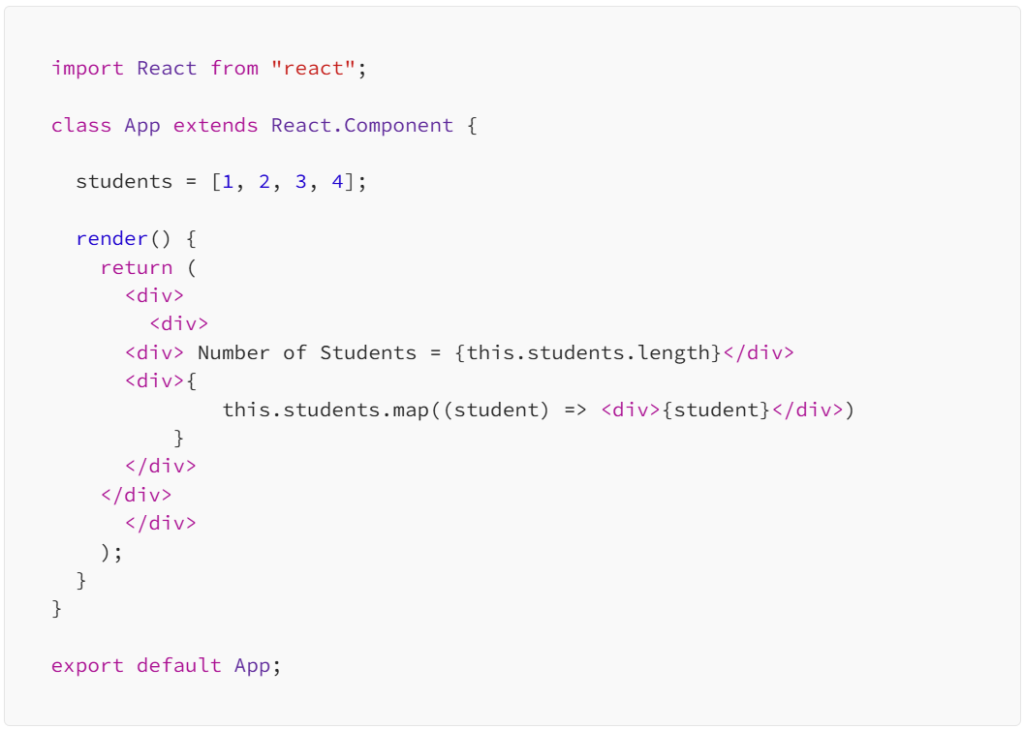
OUTPUT
Below is an output of above code snippet to render Student list item.
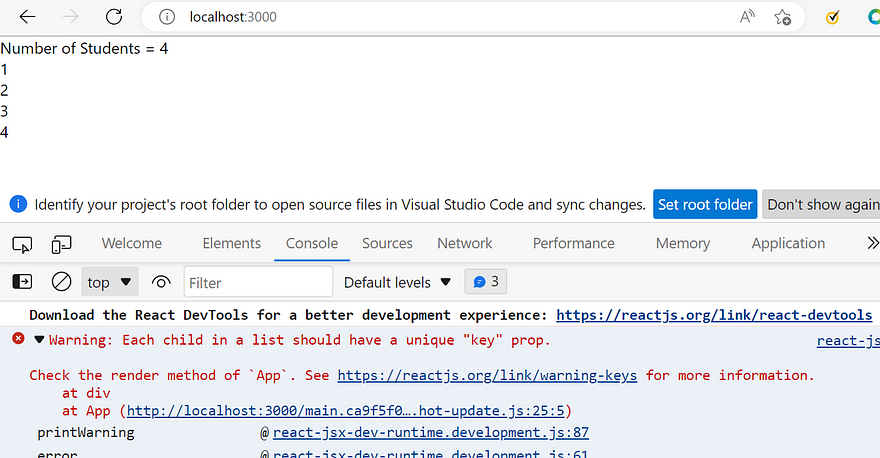
Note:
If we open browser console and verify, it is throwing an error “Warning: Each child in a list should have a unique “key” prop.” as show in above screenshot. This error is coming as we didn’t define unique element for every list item.
Provide student as key because they are unique numbers in list to every div html element while rendering Map to resolve above console log error.
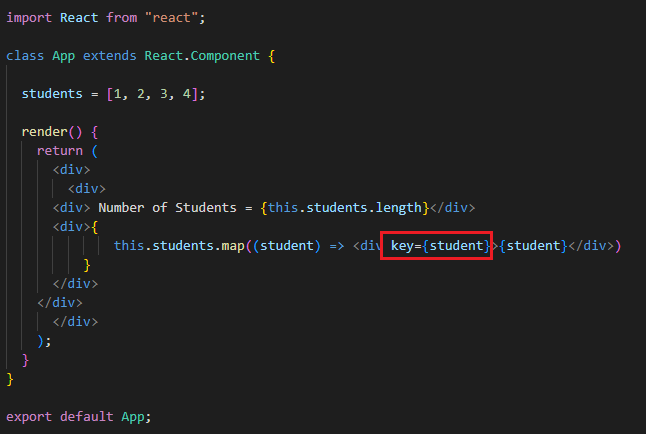
Note: student needs to be unique to avoid console log error.
OUTPUT
Below is an output of above code snippet to render Student map item without console.log error.
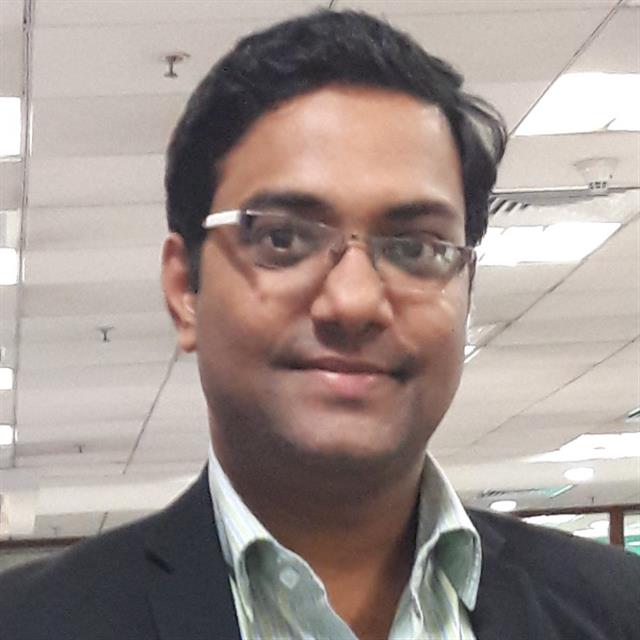
Imran Khan, Adobe Community Advisor, AEM certified developer and Java Geek, is an experienced AEM developer with over 11 years of expertise in designing and implementing robust web applications. He leverages Adobe Experience Manager, Analytics, and Target to create dynamic digital experiences. Imran possesses extensive expertise in J2EE, Sightly, Struts 2.0, Spring, Hibernate, JPA, React, HTML, jQuery, and JavaScript.