React Map
This blog will help us to work with React Map, loop and render it on the page using ES6 JavaScript map() function.
Follow below steps to create React Map component.
- Create react project using npx create-react-app practice-app command. practice-app is the custom project name.

2. Create Main.js file to create Main Class component to play with Map.
import React from 'react';
function Main () {
const students = [
{id: 1, name: 'Harry'},
{id: 2, name: 'John'},
];
return (
<div>
<div> Number of Students = {students.length}</div>
<div>{
students.map((student) => <div>{student.name}</div>)
}
</div>
</div>
);
}
export default Main;
OUTPUT
Below is an output of above code snippet to render Student Map item.
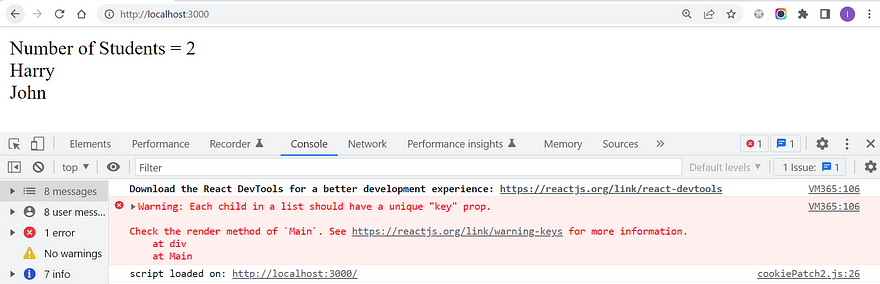
Note:
If we open browser console and verify, it is throwing an error “Warning: Each child in a Map should have a unique “key” prop.” as show in above screenshot. This error is coming as we didn’t define unique element for every Map item.
Provide student id as key to every div html element while rendering Map to resolve above console log error.
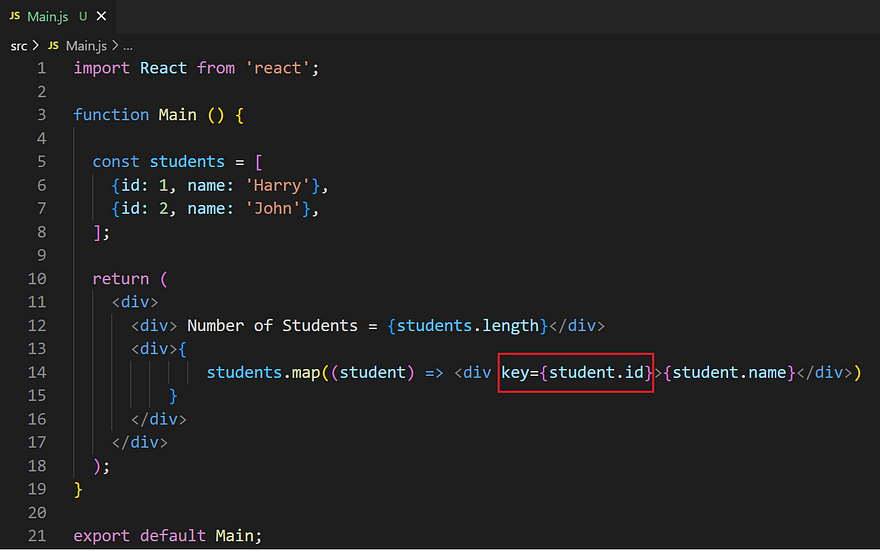
Note: student id needs to be unique to avoid console log error.
OUTPUT
Below is an output of above code snippet to render Student map item without console.log error.
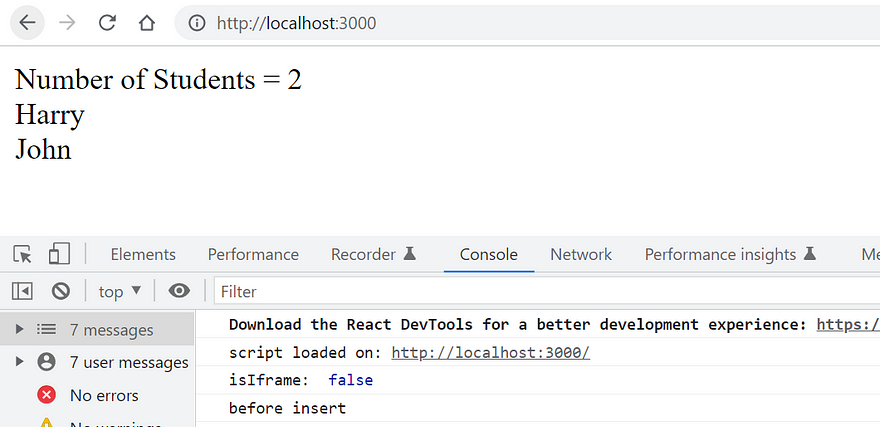
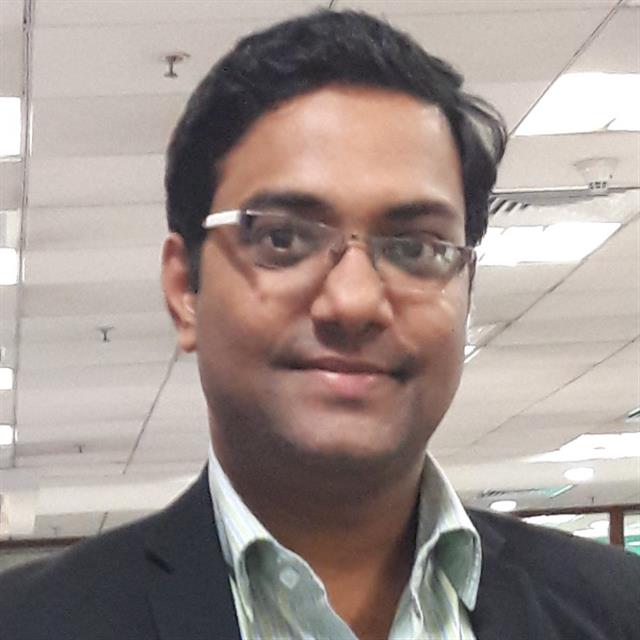
Imran Khan, Adobe Community Advisor, AEM certified developer and Java Geek, is an experienced AEM developer with over 11 years of expertise in designing and implementing robust web applications. He leverages Adobe Experience Manager, Analytics, and Target to create dynamic digital experiences. Imran possesses extensive expertise in J2EE, Sightly, Struts 2.0, Spring, Hibernate, JPA, React, HTML, jQuery, and JavaScript.