React Operators
We are going to have discussion on operators as part of this blog.
Operators are used to perform some operations between operands.
Below are the operators we are going to cover as part of this blog:
- Conditional Operators
- Logical Operator
- Ternary Operator
Conditional Operators
If else use to apply logical mathematical conditions. This will return true or false after statement execution.
Below are some conditions:
a < b : a is less than b.
a > b : a is greater than b
a == b : a is equal to b
a >= b : a is greater than equal to b
a <= b : a is less than equal to b
a != b : a does not equal to b
There are different if else statements in Java:
- if statement
- if-else statement
- nested else-if statements
Below is an example to understand various types of conditional operators such as ==, ≤ and >.
import React from 'react';
import ReactDOM from 'react-dom/client';
import Main from './Main';
function One() {
return <h3>One</h3>;
}
function Two() {
return <h3>Two</h3>;
}
function Fun(props) {
if (props.num == 1) {
return <One/>;
} else if (props.num <= 2) {
return <Two/>;
} else {
return <div>Number greater than 2</div>;
}
}
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<Fun num={3}/>
</React.StrictMode>
);
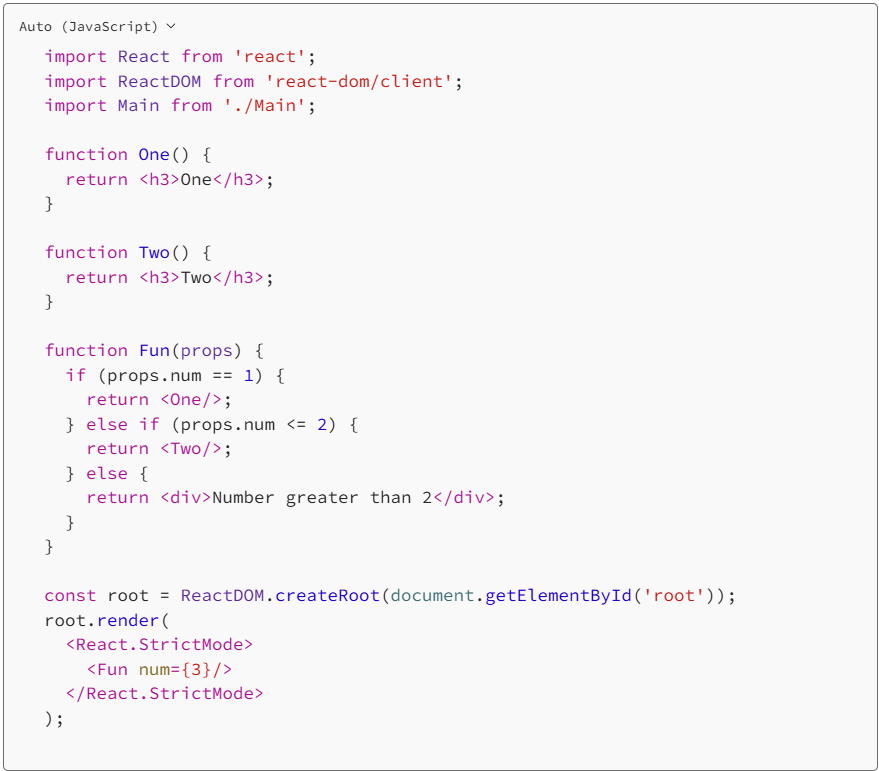
OUTPUT
Below is an output of React application for <Fun num={2}/> statement.
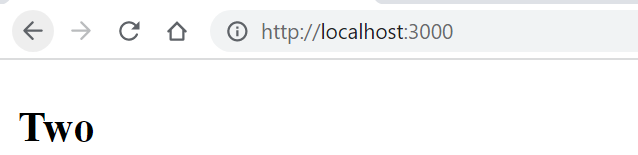
Logical Operator
Logical operator also called as a Boolean operator as it returns true or false after comparison.

Below is an example of && logical operator
import React from 'react';
import ReactDOM from 'react-dom/client';
function Fun(props) {
return (
<>
<h3>Number = {props.num}</h3>
{props.num > 1 &&
<h4>
Number greater than {1}.
</h4>
}
</>
)
}
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<Fun num={1}/>
</React.StrictMode>
);
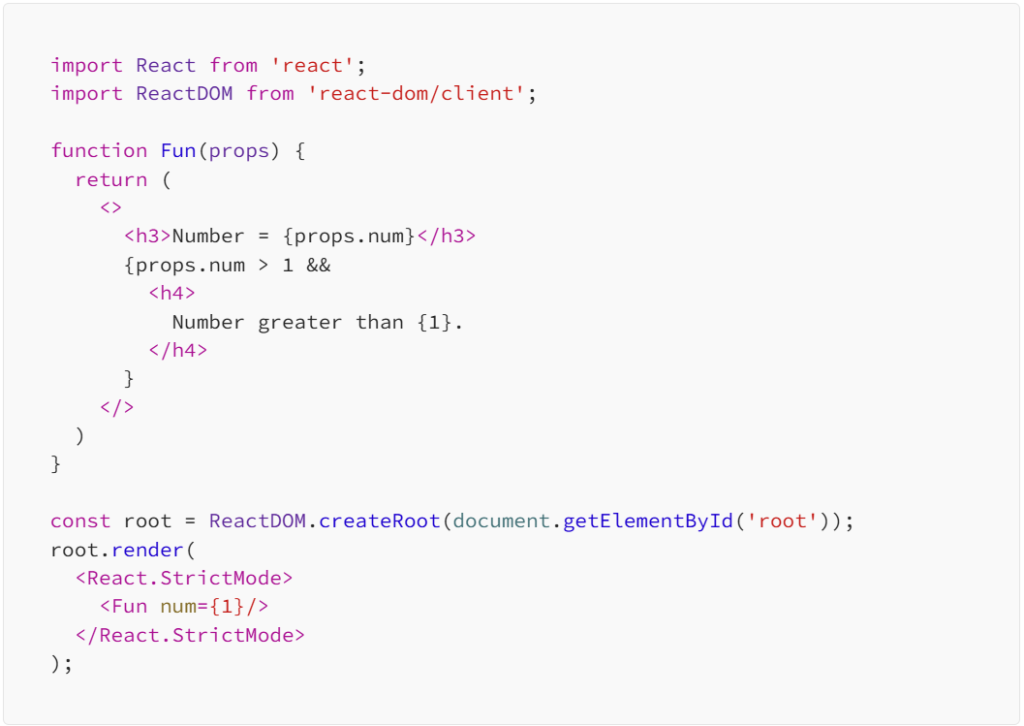
OUTPUT
Below is an output of React application for <Fun num={1}/> statement.
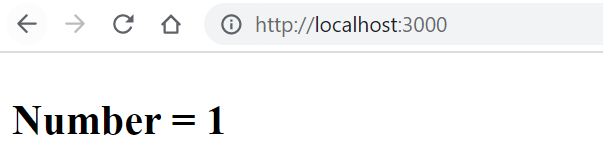
Ternary Operator
Ternary Operator evaluates a block of code based on the condition.
If condition is true, expression 1 is executed; otherwise, expression 2 is executed.
condition ? expression1 : expression2.
import React from 'react';
import ReactDOM from 'react-dom/client';
function Fun(props) {
return (
<>
{
props.num >= 1 ? <h4>One</h4> : <h4>Number greater than {1}.</h4>
}
</>
)
}
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<Fun num={1}/>
</React.StrictMode>
);
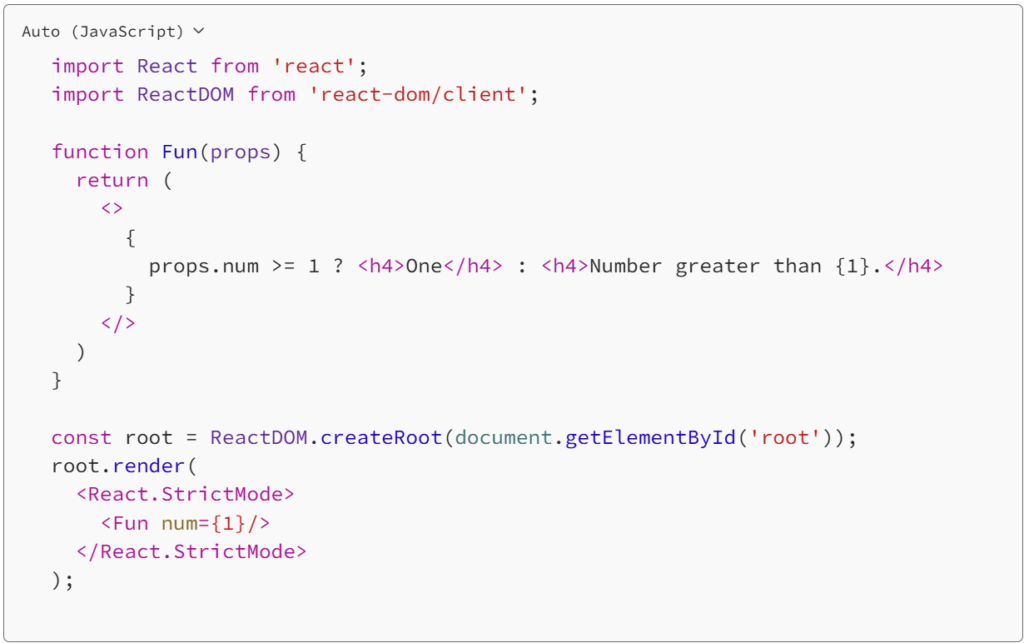
OUTPUT
- Below is an output of React application for <Fun num={1}/> statement.
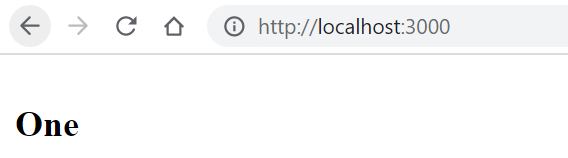
2. Below is an other variation of an output of React application for <Fun num={2}/> statement.
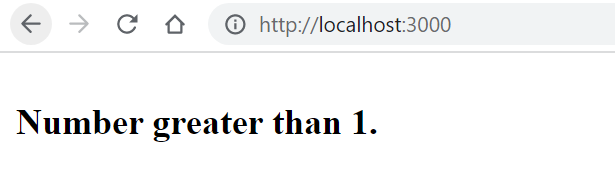
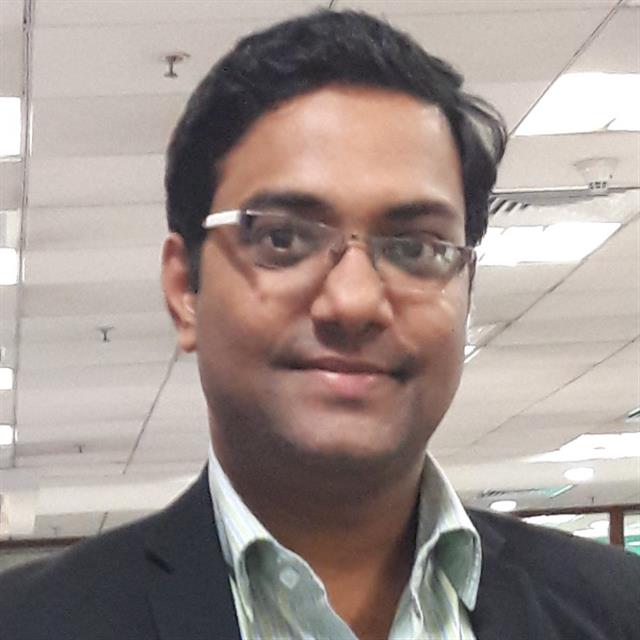
Imran Khan, Adobe Community Advisor, certified AEM developer and Java Geek, is an experienced AEM developer with over 12 years of expertise in designing and implementing robust web applications. He leverages Adobe Experience Manager, Analytics, and Target to create dynamic digital experiences. Imran possesses extensive expertise in J2EE, Sightly, Struts 2.0, Spring, Hibernate, JPA, React, HTML, jQuery, and JavaScript.