React CSS
There are multiple ways to write CSS which helps us to include style.
Inline Style CSS
Below is an example of inline styling.
In JSX, inline style follows camel case letter. e.g. background-color will be written as backgroundColor, padding-left as paddingLeft, etc.
import React from "react";
class App extends React.Component {
render() {
return (
<div>
<h1 style={{color: "green", backgroundColor: "yellow"}}>Hello World !!!</h1>
</div>
);
}
}
export default App;
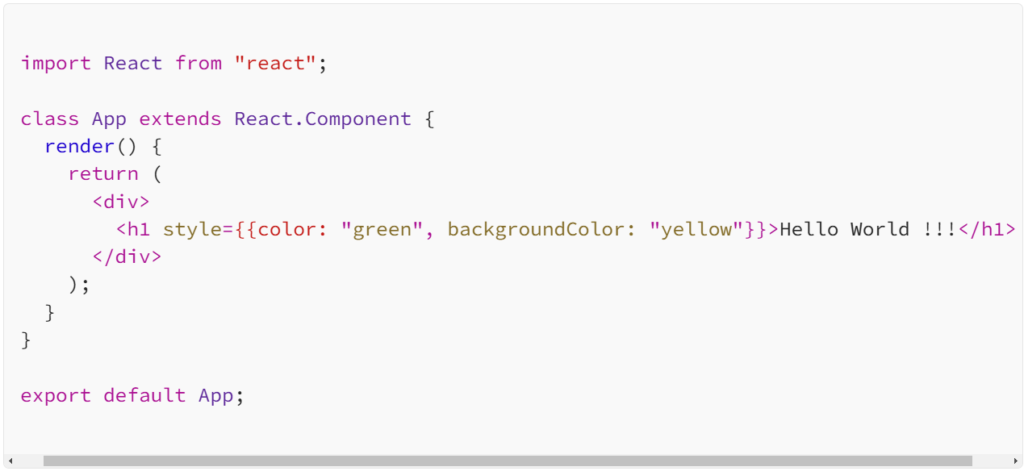
OUTPUT:
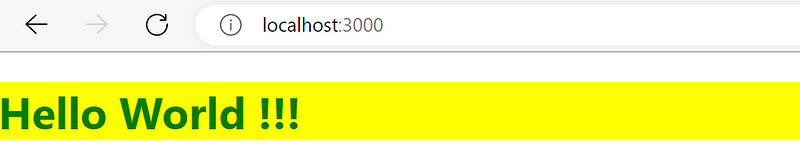
CSS Object
we can write CSS in the form of object and apply to an element as shown below.
Below is an example of reactCss object having color and backgroundColor as CSS.
import React from "react";
class App extends React.Component {
reactCss = {
color: "green",
backgroundColor: "yellow"
};
render() {
return (
<div>
<h1 style={this.reactCss}>Hello World !!!</h1>
</div>
);
}
}
export default App;
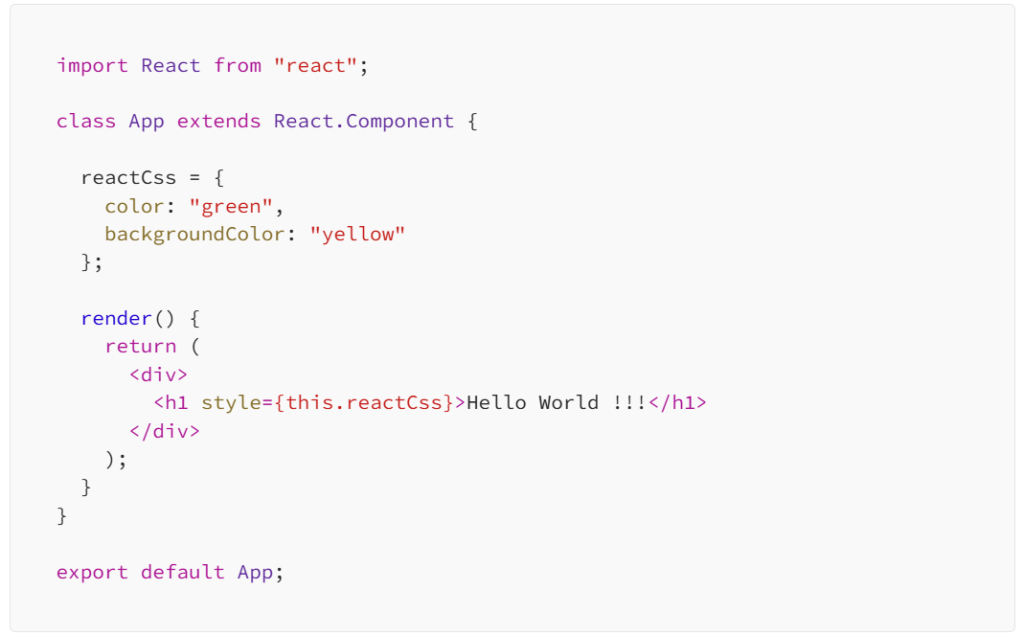
OUTPUT:
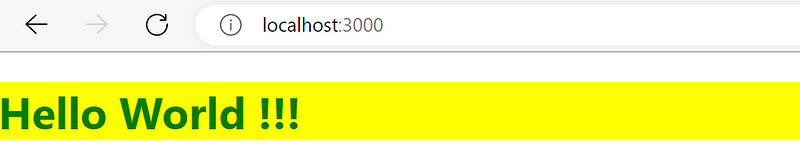
External CSS
Below is an External CSS index.css file.
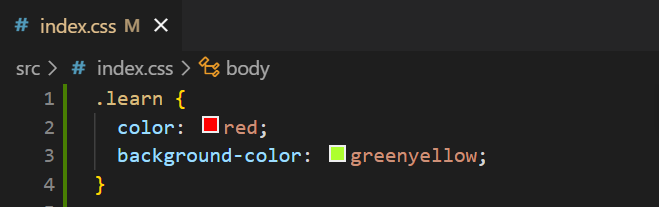
Below is the component consuming learn class CSS written in index.css file.
import React from "react";
import "./index.css"
class App extends React.Component {
render() {
return (
<div>
<h1 class="learn">Hello World !!!</h1>
</div>
);
}
}
export default App;
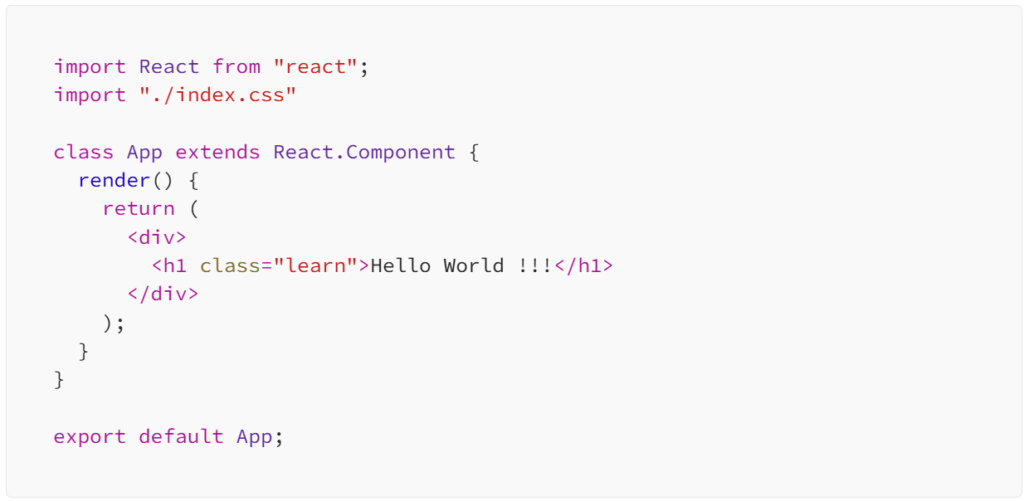
OUTPUT:

CSS Module
CSS module is the another best way to write and consume styles in react.
Create a CSS module as learn.module.css having below styles:
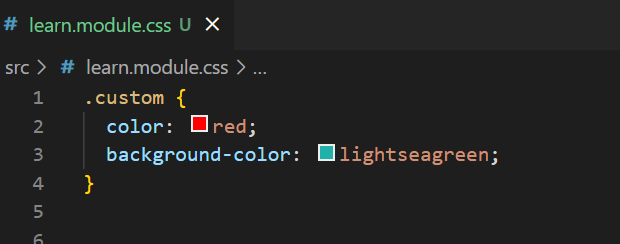
Import above create learn.module.css file in component as shown below and consume using className attribute.
import React from "react";
import styles from "./learn.module.css";
class App extends React.Component {
render() {
return (
<div>
<h1 className={styles.custom}>Hello World !!!</h1>
</div>
);
}
}
export default App;
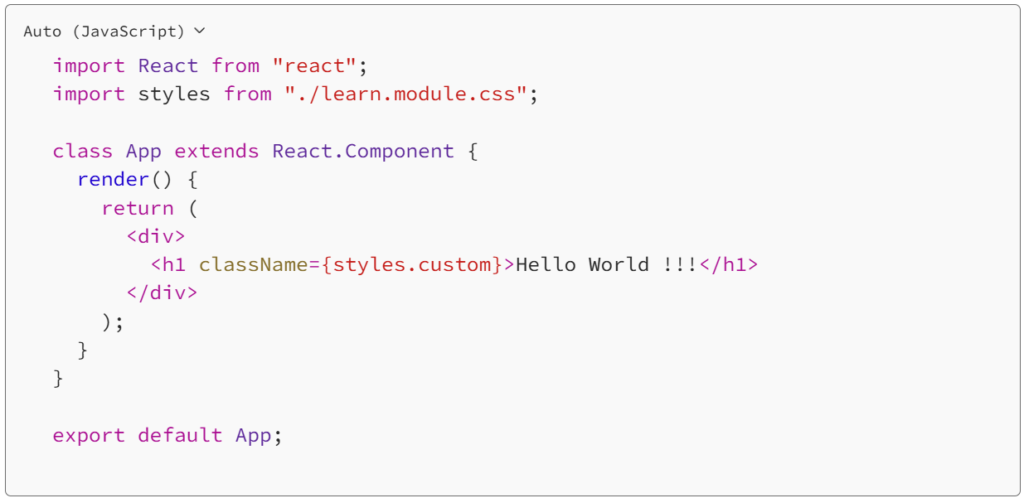
OUTPUT:
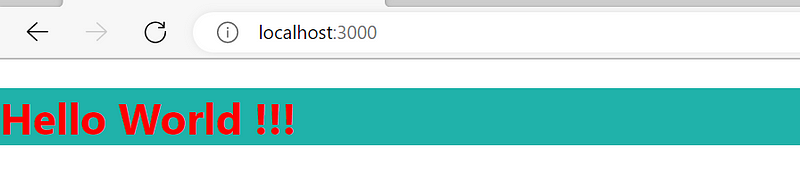
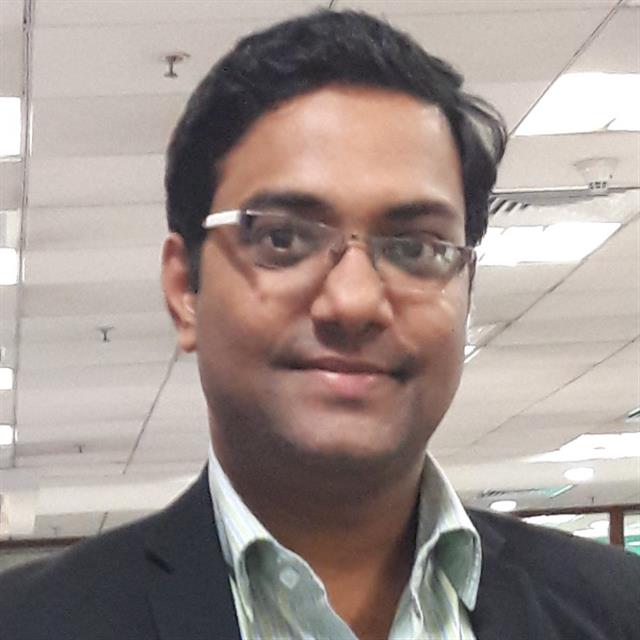
Imran Khan, Adobe Community Advisor, AEM certified developer and Java Geek, is an experienced AEM developer with over 11 years of expertise in designing and implementing robust web applications. He leverages Adobe Experience Manager, Analytics, and Target to create dynamic digital experiences. Imran possesses extensive expertise in J2EE, Sightly, Struts 2.0, Spring, Hibernate, JPA, React, HTML, jQuery, and JavaScript.