JSX
JSX is also called as JavaScript XML.
Let’s try to understand all about JSX and where it gets consume within React components.
Functional and Class component use render() method to load HTML which called as JSX. In simple terms, JSX allow us to load write an HTML code with render() method as highlighted below:
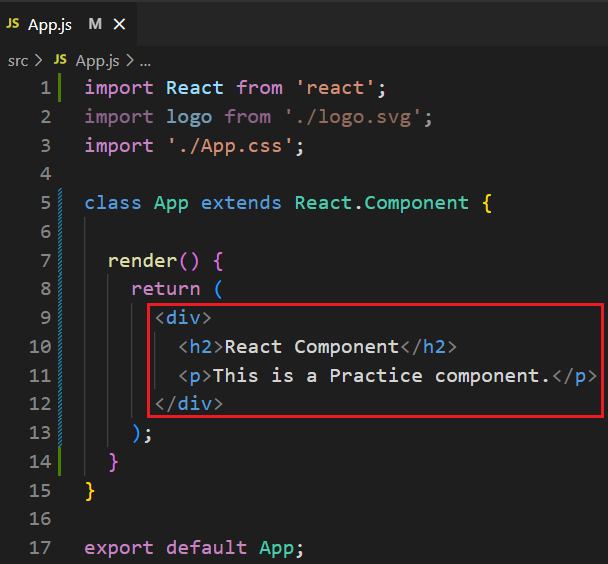
Below is the JSX example to load html code with the help of render() method:
import React from 'react';
import ReactDOM from 'react-dom/client';
const jsxCode = <h3>This is JSX code !!!</h3>;
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(jsxCode);
Below is an example of code not using JSX code as React.createElement to create a DOM element:
import React from 'react';
import ReactDOM from 'react-dom/client';
const jsxCode = React.createElement('h3', {}, 'This is JSX code !!!');;
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(jsxCode);
OUTPUT:

Expression using JSX
Below is an example of expression as part of JSX using curly braces as {}
import React from 'react';
import ReactDOM from 'react-dom/client';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<h3>Sum of 3 + 2 is {3 + 2}</h3>;
</React.StrictMode>
);
Multiple HTML elements
React doesn’t allow us to write multiple html elements within render() method and gives below error.
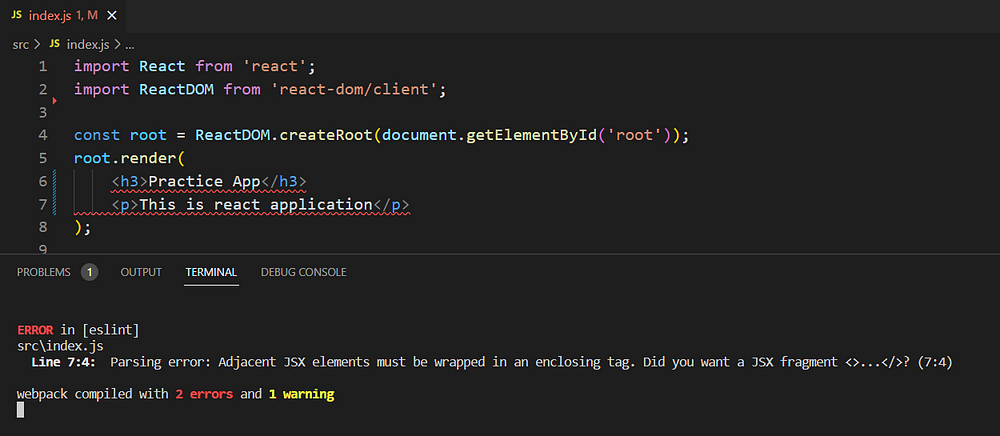
To resolve above issue, it is always require to write a root element as highlighted below at line number 6:
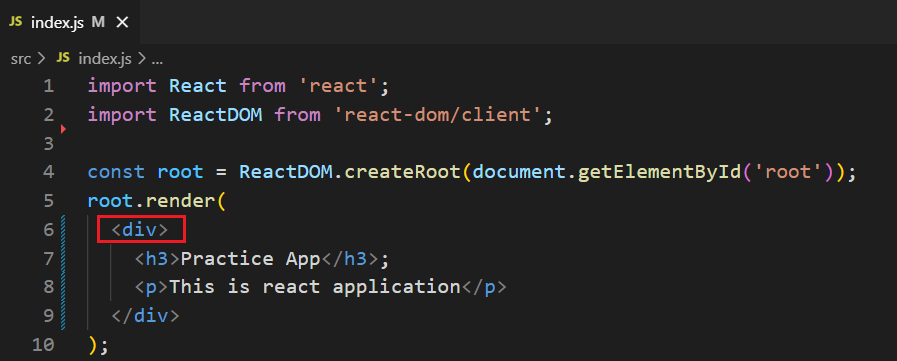
Note:
We can use <React.Fragment> or <> to resolve above adjacent JSX elements issue. Fragment takes less memory as they don’t create an extra tag in the DOM.
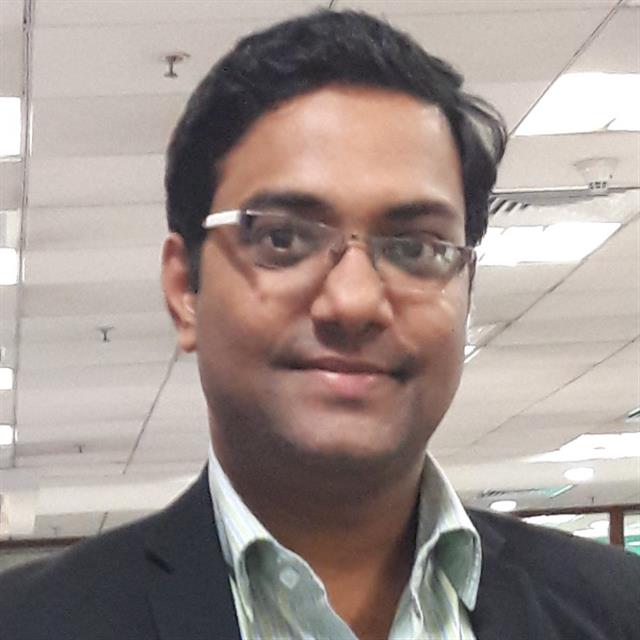
Imran Khan, Adobe Community Advisor, certified AEM developer and Java Geek, is an experienced AEM developer with over 12 years of expertise in designing and implementing robust web applications. He leverages Adobe Experience Manager, Analytics, and Target to create dynamic digital experiences. Imran possesses extensive expertise in J2EE, Sightly, Struts 2.0, Spring, Hibernate, JPA, React, HTML, jQuery, and JavaScript.