Fragment
Return or render function don’t allow us to return multiple tag elements. Fragment allow us to have one root tag element for multiple child tags as shown below.
Not using Fragment will always create an extra DOM node.

In place of <React.Fragment> we can use other tag elements also such as div, section, table, etc.
Using tag other than Fragment will create an extra tag in HTML DOM as shown above.
There is no extra tag got created with the name fragment as we can verify in above screen shot.
Follow below steps to see Fragment usage.
- Create react project using npx create-react-app practice-app command. practice-app is the custom project name.

2. Paste below code inside index.js file.
import React from 'react';
import ReactDOM from 'react-dom/client';
class Practice extends React.Component {
render() {
return (
<React.Fragment>
<h2>React Title</h2>
<p>This is a descriotion</p>
</React.Fragment>
);
}
}
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<Practice num={1}/>
</React.StrictMode>
);
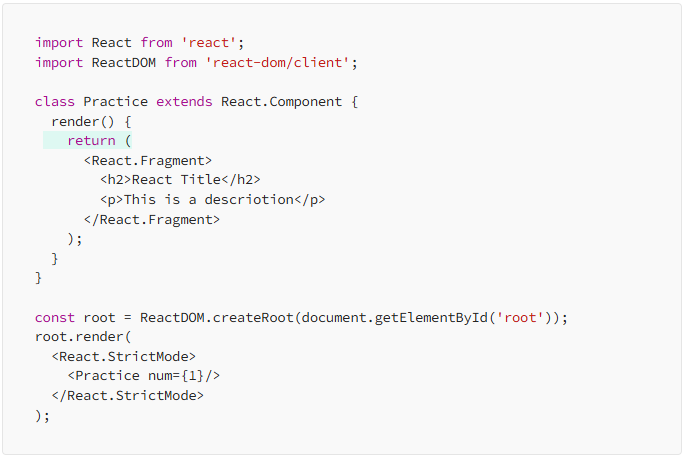
OUTPUT
Below is an output of above code snippet.

Shorthand Fragment
Shorthand Fragment allow us to write empty tag <> in place of <React.Fragment>
import React from 'react';
import ReactDOM from 'react-dom/client';
class Fun extends React.Component {
render() {
return (
<>
<h2>React Title</h2>
<p>This is a descriotion</p>
</>
);
}
}
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<Fun num={1}/>
</React.StrictMode>
);
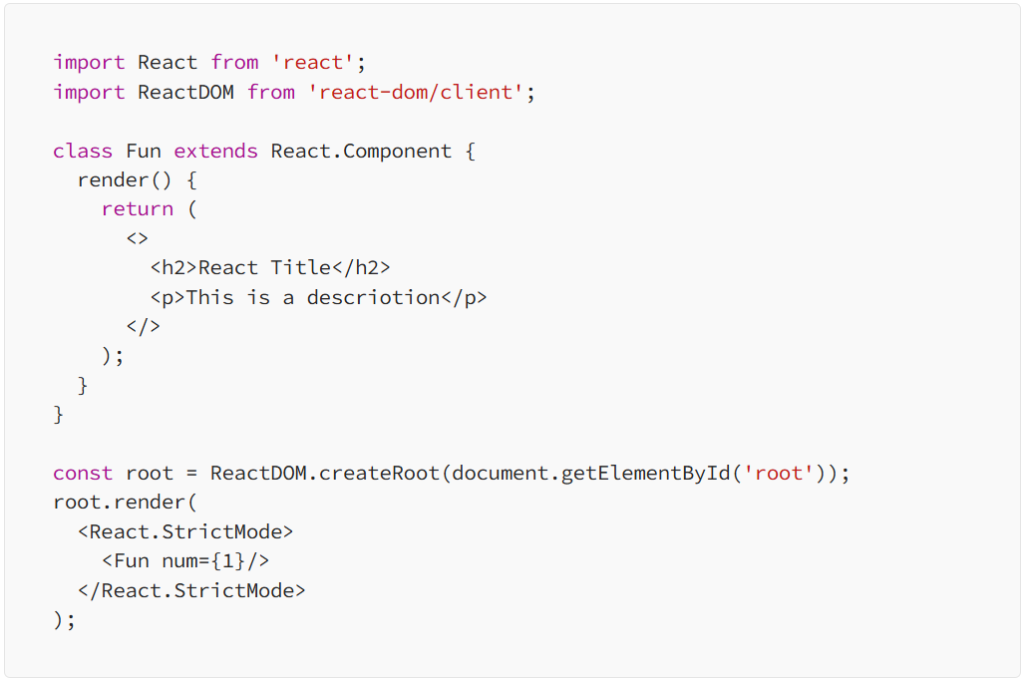
Note:
Shorthand fragment <> don’t allow to have key attribute. <React.Fragment key=’abc’> is a valid statement.
Advantage
Fragment takes less memory as they don’t create an extra tag in the DOM.
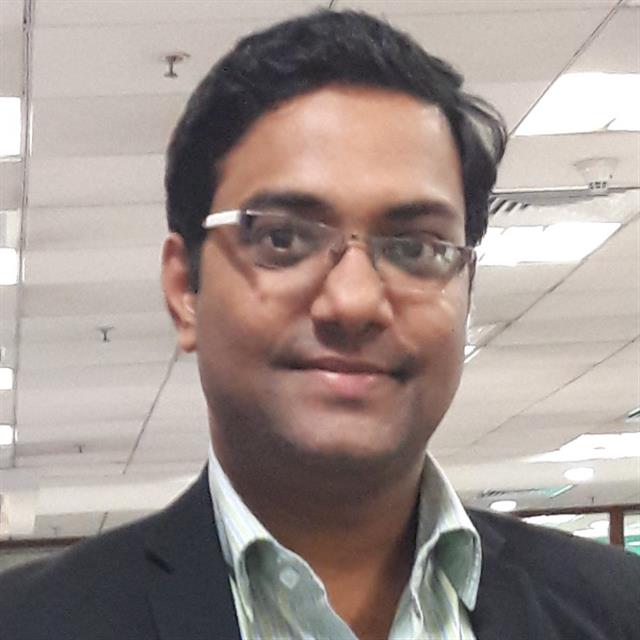
Imran Khan, Adobe Community Advisor, certified AEM developer and Java Geek, is an experienced AEM developer with over 12 years of expertise in designing and implementing robust web applications. He leverages Adobe Experience Manager, Analytics, and Target to create dynamic digital experiences. Imran possesses extensive expertise in J2EE, Sightly, Struts 2.0, Spring, Hibernate, JPA, React, HTML, jQuery, and JavaScript.