Bean Definition, Life Cycle, Inheritance, Abstract and BeanPostProcessor
This is a one complete single guide to understand things all about Spring bean.
Spring Bean is a normal pojo Java class with setter and getter methods which get initialized by Spring Container.
It gets call as Spring bean if it is initialized by Spring container.
On high level Spring bean is all about three things.
1. Spring bean definition
2. Spring bean scopes
3. Spring bean life cycle.
Spring bean metadata Creation:
It can be configured using below methods:
1. XML based configuration
2. Annotation based configuration
3. Java based configuration.
In this blog we will talk about first option XML based configuration.
There are multiple attributes which are defined for <bean> tag:
class: This attribute will be looking for complete class name with package.
id: This will help us to create an instance of a class, similar to new keyword in Java. It is also called as bean identifier
name: This will work as an instance name defined with new keyword in Java or also called as bean identifier. name helps us to create an alias for bean class.
scope: This will define the scope of object. Spring supports singleton, prototype, request, session and global-session as a scope.
constructor-arg: This will be use to inject the dependency using constructor arguments.
autowire:
This will be use for dependency injection.
There are four types of auto wiring mode as no, byName, byType, constructor
no → This is Spring’s default, also called as No automagical wiring. ref tag element uses to define bean references in xml file. autowire=default is equivalent to autowire=no
byName → Properties of the autowired bean will be wired by searching for bean with same name/id in the configuration file. If such a bean is found it is injected, properties for which no matching bean is found will remain unwired which may later result in error.
byType → It will look for bean having compatible property’s type.
constructor → Wiring will be done one the basis of constructor. It will look for the bean with matching constructor argument.
init-method: This will call custom method will get invoke just after setting bean properties. The method must have no arguments, but may throw any exception.
destroy-method: This will call custom destroy method defined having no arguments. This function can throw an exception.
lazy-init: It is used for IoC container to create a bean instance when it is first requested, rather than at the startup of the application or on load of config file.
Note: We have multiple inline examples for all above attributes.
Below are some of the example to define bean attribute.

Bean Scope
As discussed above scope is an attribute of bean tag. Bean tag helps us to create an instance of bean or pojo class.
Spring supports singleton, prototype, request, session and global-session as bean scopes.
Singleton: It will create an single instance per Ioc container.
request: This will be there for a single HTTP request.
session: This will be valid for an HTTP session.
prototype: Bean can have any number of objects.
global-session: This will be valid for global HTTP session.
Singleton Scope
As we all aware about singleton design pattern. In singleton pattern make sure only object will get create of class in an application. Creation of second object will refer to the first one.
Create a person Person class with name variable and setter method to set value of name variable. Create one getter method to print name variable value.

Add a bean tag having a scope attribute with a value of singleton, which allows for only one object per IoC container.

In DemoApplication.java class inside main method, create an object as person and set a name variable value as “John”.
Create another object as person2 at line number 2 and calling getName() method will return “John” as it will refer to the previous object.

OUTPUT:
Name = John
Name = John
Prototype Scope
This is a scope which will always return a new instance of that class.
Create a person Person class with name variable and setter method to set value of name variable. Create one getter method to print name variable value.

Add a bean tag having a scope attribute with a value of singleton, which allows for only one object per IoC container.

In DemoApplication.java class inside main method, create an object as person and set a name variable value as “John”.
Create another object as person2 at line number 2 and calling getName() method will return null as we didn’t set any value person2 object name variable.

OUTPUT:
Name = John
Name = null
Bean Life Cycle
The Spring life cycle has two init and destroy methods.
The init method will get called just after the initialization of bean properties to manipulate data.
The destroy method gets called when a bean is no longer required and the object is ready to decompose. This method is used as a cleanup activity.
Create Person.java class with init() and destroy() method as shown below.

Create a bean tag element as mentioned below at line number 8. Declare the init-method and destroy-method attributes’ values as init and destroy, respectively, as defined in the Person.java class.

Create an instance of Person class using ClassPathXmlApplicationContext class at line number 12.

Run DemoApplication.java will show below output.
OUTPUT:
init() method called
Print name
destroy() method called
If we have multiple classes that have init and destroy methods defined.
We can define default-init-method and default-destroy-method for all beans at once, as shown below, in place of writing init-method and destroy-method for every bean tag element.

Bean Inheritance
Spring can build a child and parent inheritance relationship using SpringConfig.xml using parent keyword as shown in below diagram.
In below inline screenshot, we are creating a parent child relationship in between Type and car class. Type will be a base or parent class and Car will be child or derived class.
Created two objects using id attribute as type and car for com.example.demoType and com.example.demo.Car class respectively.
Declared parent keyword attribute value as type highlighted below red in color to make it as parent class of car.

Create a class name as Type.java with variable name as model with setter method.

Create a class Car.java and declare property named as name and model. model is an existing property in class Type.java also.

Declared parent keyword attribute value as type highlighted below red in color to make it as parent class of car.
Note: model property and setter is defined in both Type.java and Car.java class.
model variable assigned a value as Sedan only in Type.java class.
Car.java class will automatically inherit model variable value from Type.java class due to inheritance using parent keyword assigned value as type as shown below.
parent=”type”.

Run main() method from DemoApplication.java class. car.printCarModel() will get value printed as Sedan using Type.java class using inheritance.

OUTPUT:
Model = Sedan
Model = Sedan
Name = Honda
abstract attribute
Having the abstract keyword value be true will make a class abstract, similar to how we declare a class as abstract using the abstract keyword. Making a class abstract will not allow you to create its object or an instance.

OUTPUT:

Note: Type.java class will give above error on instance creation as Type bean declared as abstract.
Remove below highlighted code red in color will resolve build / code failure.

OUTPUT:
Name = Honda
BeanPostProcessor
BeanPostProcessor interface have two methods postProcessBeforeInitialization()
and postProcessAfterInitialization().
These methods will get called on every bean initialization.
Create Car.java class with init and destroy method.

Type.java class implements BeanPostProcessor interface. Override postProcessBeforeInitialization()
and postProcessAfterInitialization()
methods.
Define init() and destroy() method for Type.java class.

Declaring the default-init-method and default-destroy-method attribute values as true in the beans tag will call the init() and destroy() methods of every bean class.

OUTPUT:
Type init() method called
Before Initialization = car
Car init() method called
After Initialization = car
Name = Honda
Car destroy() method called
Type destroy() method called
Bean Has-A relationship
In Java, Has-A relationship is also called as composition. This means class is having a reference of another class.

Create Type.java class having model as field and its getter setter.

In below screenshot Car.java class is having a reference of above Type.java class which shows Has-A relationship highlighted in red color.

Inject Type.java bean class with Sedan as model value within Car.java bean class.

Create an instance of Car class using ApplicationContext and run it as Java application.

Car bean class is calling getType() method which is internally calling getModel() method as shown below.

OUTPUT:
Model = Sedan
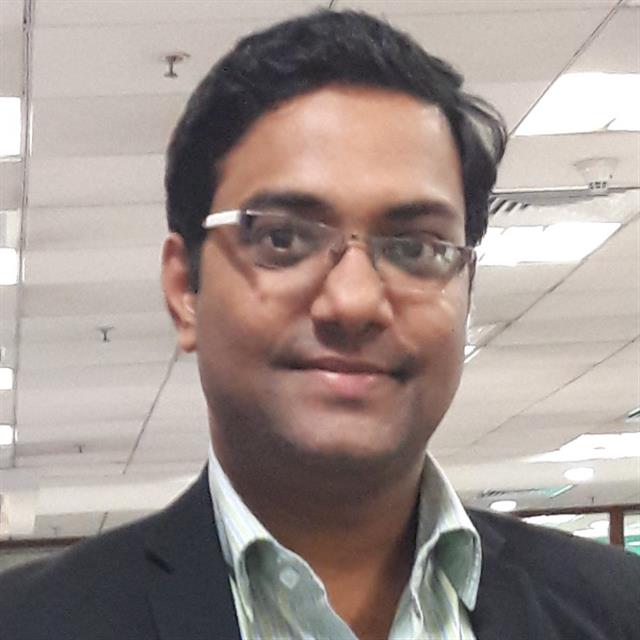
Imran Khan, Adobe Community Advisor, AEM certified developer and Java Geek, is an experienced AEM developer with over 11 years of expertise in designing and implementing robust web applications. He leverages Adobe Experience Manager, Analytics, and Target to create dynamic digital experiences. Imran possesses extensive expertise in J2EE, Sightly, Struts 2.0, Spring, Hibernate, JPA, React, HTML, jQuery, and JavaScript.